Configuring Automated Testing with Selenium and Java in GitLab CI Pipeline with Allure Reporting
Introduction
In today’s world of rapid software development, continuous integration (CI) and automated testing have become essential components of a successful software development process. These practices help maintain high code quality, increase collaboration among team members, and speed up the implementation of changes. Additionally, automated testing and CI allow developers to catch issues early in the development cycle, reducing the time and cost of fixing bugs.
In this article, I will demonstrate how to configure automated testing using Selenium and Java within a GitLab CI pipeline, a popular CI/CD platform that offers a wide range of features for managing code, builds, and deployments. We will also explore the generation of Allure reports, which provide comprehensive test result visualizations and insights, allowing teams to quickly identify areas of improvement.
The article will guide you through the following steps:
- Project setup: Preparing your Java project with the necessary Selenium and Allure dependencies using Maven or Gradle.
- GitLab CI configuration: Creating a .gitlab-ci.yml file to define tasks, stages, and the environment for your pipeline.
- Writing and running Selenium tests: Developing sample Selenium tests using JUnit 5 and Selenium WebDriver.
- Viewing and analyzing Allure reports: Accessing and analyzing the generated Allure report to effectively evaluate test results.
- By the end of this article, you will have a solid understanding of how to implement a GitLab CI pipeline for automated testing with Selenium and Java, and how to utilize Allure reports to enhance your testing process.
1.Project Setup
A) Maven – First, make sure your Java project is set up with tools like Maven or Gradle for automated building. A sample pom.xml (Maven) file should include the necessary Selenium and Allure dependencies:
<dependencies>
<!-- Selenium WebDriver -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
<!-- Allure integration -->
<dependency>
<groupId>io.qameta.allure</groupId>
<artifactId>allure-junit5</artifactId>
<version>2.17.2</version>
</dependency>
</dependencies>
B) Gradle – Add the necessary dependencies to your build.gradle file:
plugins {
id 'java'
id 'io.qameta.allure' version '2.8.1'
}
repositories {
mavenCentral()
}
dependencies {
// Selenium WebDriver
implementation 'org.seleniumhq.selenium:selenium-java:3.141.59'
// Allure integration
testImplementation 'io.qameta.allure:allure-junit5:2.17.2'
}
allure {
autoconfigure = true
useJUnit5 {
version = '2.17.2'
}
}
2. GitLab CI Configuration
A) Maven – add a .gitlab-ci.yml file to the root directory of your project. In this file, we will define all tasks and the environment in which they will run. A sample configuration looks like this:
image: maven:3.8.2-openjdk-11
cache:
paths:
- .m2/repository
stages:
- build
- test
- report
build:
stage: build
script:
- mvn clean compile
test:
stage: test
script:
- mvn test
artifacts:
when: always
paths:
- target/allure-results
expire_in: 1 day
allure-report:
image: "franela/allure-docker:latest"
stage: report
script:
- allure generate --clean target/allure-results -o target/allure-report
artifacts:
when: always
paths:
- target/allure-report
expire_in: 1 day
B) Gradle – modify your .gitlab-ci.yml file to use the Gradle commands instead of Maven:
image: gradle:7.3.3-jdk11
cache:
paths:
- .gradle/caches
stages:
- build
- test
- report
build:
stage: build
script:
- gradle clean compileJava
test:
stage: test
script:
- gradle test
artifacts:
when: always
paths:
- build/allure-results
expire_in: 1 day
allure-report:
image: "franela/allure-docker:latest"
stage: report
script:
- allure generate --clean build/allure-results -o build/allure-report
artifacts:
when: always
paths:
- build/allure-report
expire_in: 1 day
3. Configuration Explanation
A) Maven – In the example .gitlab-ci.yml file above, we use the Docker image maven:3.8.2-openjdk-11, which contains Maven and JDK 11. We define three stages (stages): build, test, and report.
In the build stage, we compile our code using the mvn clean compile command. In the test stage, we run the Selenium tests using the mvn test command. After the tests are executed, the results are stored in the target/allure-results directory. We use the artifacts section to store the test results, which will be used in the next stage.
In the report stage, we generate the Allure report. We use the Docker image franela/allure-docker:latest, which contains the Allure tool. The script runs “allure generate”, which converts the test results from the target/allure-results directory into an Allure report stored in the target/allure-report directory. We also use the artifacts section to store the generated Allure report, which can then be accessed and analyzed by the team.
B) Gradle – In this example, we have updated the build.gradle file with the required Selenium and Allure dependencies. We have also configured the Allure plugin for Gradle, which enables Allure reporting during test execution.
Additionally, we have modified the .gitlab-ci.yml file to use the Gradle commands for building and testing the project. The image field now uses gradle:7.3.3-jdk11, which contains Gradle and JDK 11. The rest of the pipeline remains the same, with the build, test, and report stages performing the respective tasks using Gradle commands.
4. Writing and Running Selenium Tests
Now that the CI pipeline is set up, let’s create some sample Selenium tests. We will use the JUnit 5 testing framework in conjunction with Selenium WebDriver. Here is a basic example of a test class:
import org.junit.jupiter.api.*;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class SampleSeleniumTest {
private WebDriver driver;
@BeforeEach
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
}
@Test
public void pageTitleTest() {
driver.get("https://www.example.com");
String title = driver.getTitle();
assertEquals("Example Domain", title);
}
@AfterEach
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
In this example, we have tested the page title of “https://www.example.com”. In the setUp() method, we initialize the Chrome WebDriver, and in the tearDown() method, we close the WebDriver.
5. Viewing and Analyzing Allure Reports
After the GitLab CI pipeline has successfully completed its task, you can access the generated Allure report in the pipeline’s artifacts section. Download the Allure report and open the index.html file in a web browser to view the results.
The Allure report provides a comprehensive overview of your test results, including pass/fail status, execution time, and other relevant information. It also offers various visualizations and filtering options to help you analyze the test results more effectively.
Summary
In this article, we have demonstrated how to configure a GitLab CI pipeline to run automated tests with Selenium and Java and generate Allure reports after the tests have been completed. This setup enables teams to maintain high code quality, streamline the development process, and quickly identify any issues that arise during testing. By incorporating automated testing and continuous integration practices, you can significantly improve your software development workflow.
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us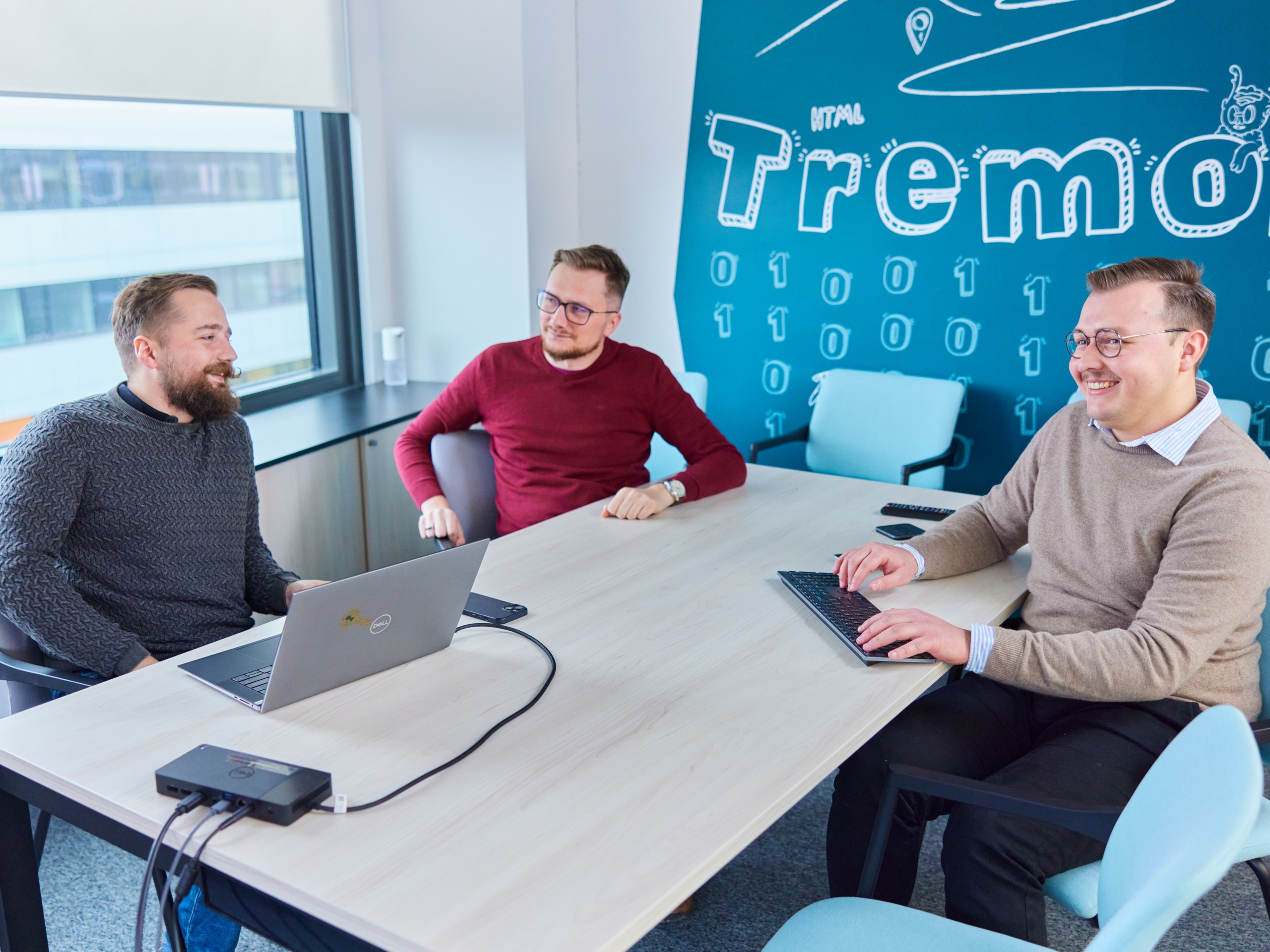
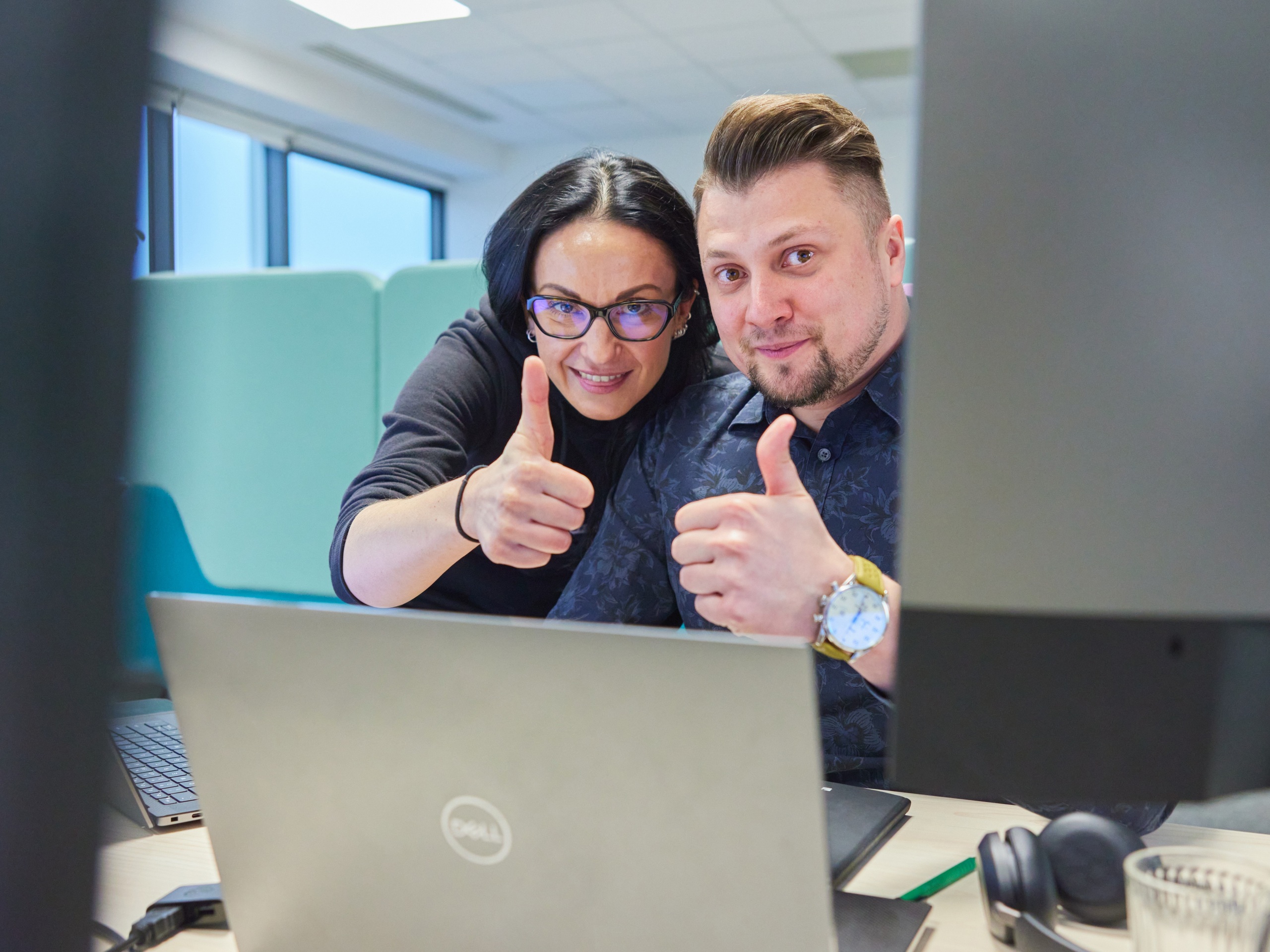
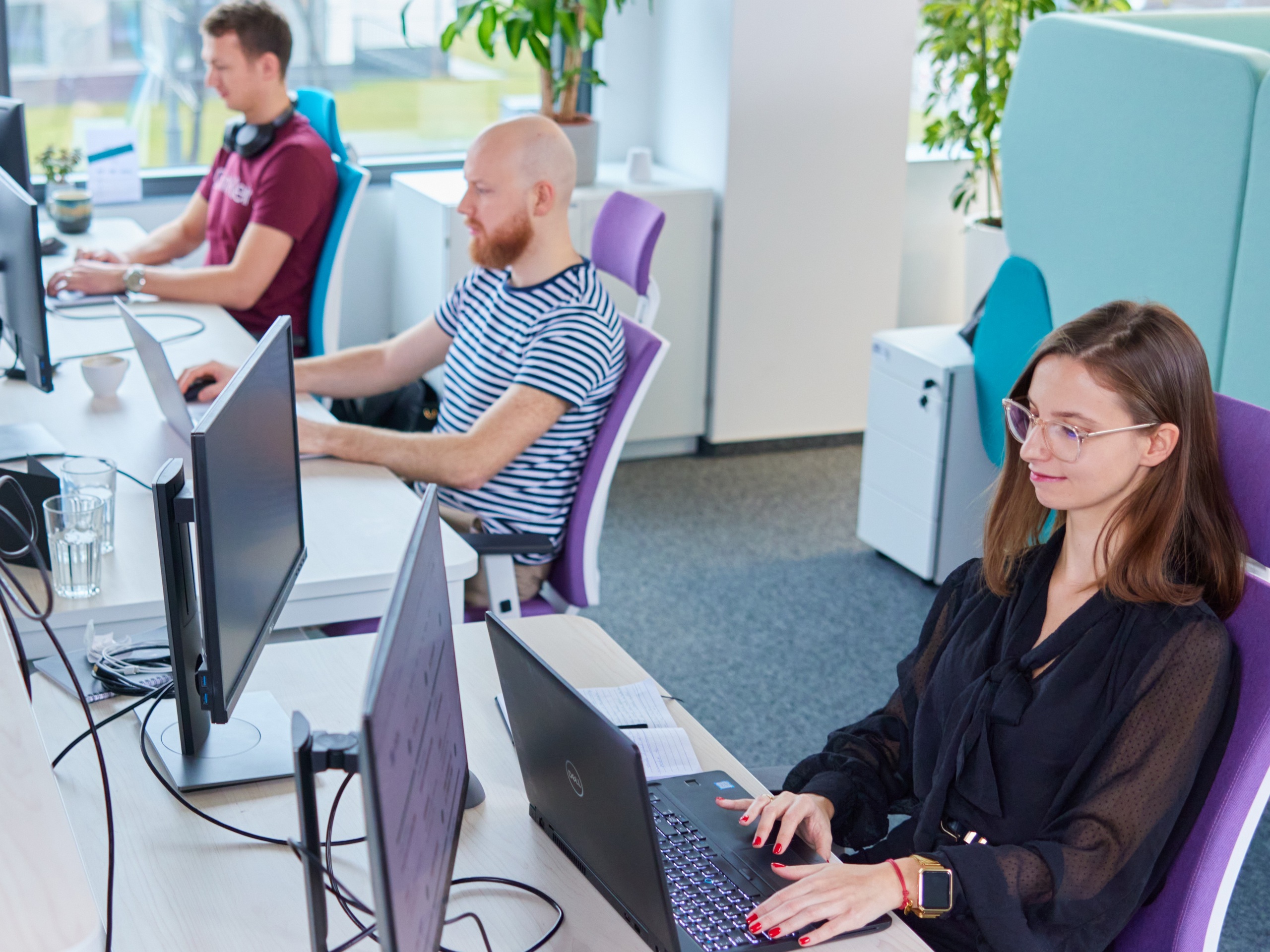
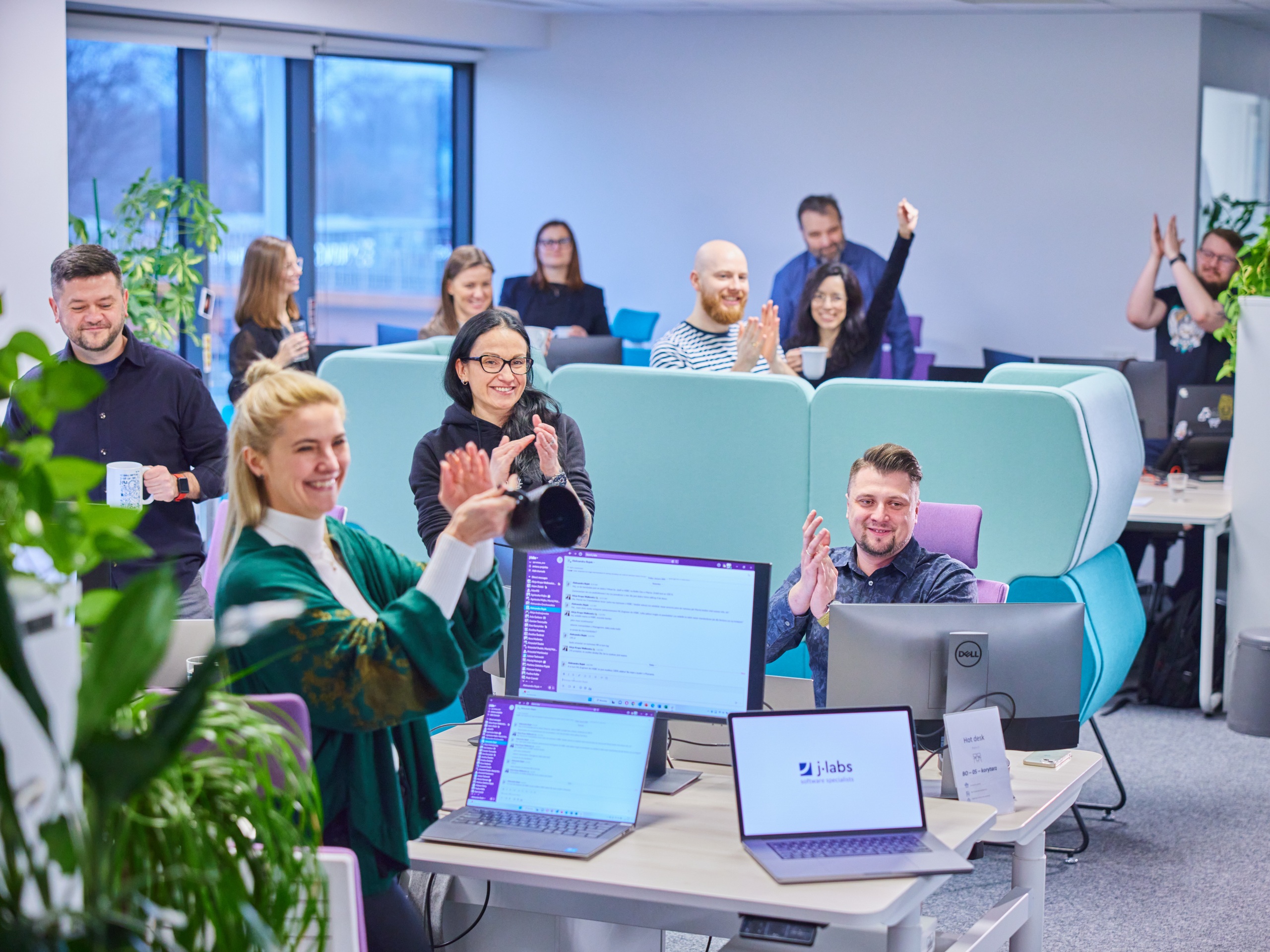