Practical guide to gRPC in Java
We have updated this text for you!
Update date: 26.12.2024
Author of the update: Adam Olszewski
gRPC is an open-source, language-agnostic RPC framework, developed by Google in 2015, based on their internal framework Stubby, which powers billions of requests per second. Since its inception, gRPC has seen continuous improvements, with significant updates in both its features and ecosystem support.
Comparison with SOAP and Rest
While gRPC shares similarities with SOAP (due to its contract-first nature and mandatory message definitions), it offers several key advantages over both SOAP and REST:
- Simpler Contracts: gRPC uses protocol buffers (.proto files), a much simpler, faster, and more efficient format than XML-based WSDL used in SOAP.
- Better Performance: Protocol Buffers (protobuf) is a binary format that is more compact and faster than both JSON (used in REST) and XML (used in SOAP). This is especially important in high-performance applications.
- Streaming Support: gRPC natively supports streaming, both client-to-server and server-to-client, whereas REST often requires additional layers to achieve this.
- Built-in Deadlines and Cancellations: gRPC allows developers to set deadlines and cancel RPCs, which is harder to achieve with REST.
- Code Generation: gRPC tools automatically generate client and server code, reducing boilerplate code and enhancing productivity.
Protocol Buffers (protobuf) Under the Hood
Protocol Buffers (protobuf) is a method of serializing structured data, which gRPC uses for efficient communication between services. Protobuf is much smaller and faster than JSON or XML, thanks to its binary format. Each message in protobuf consists of a series of key-value pairs.
Here’s an updated example of a Car message definition:
message Car {
int32 id = 1;
string name = 2;
}
Protobuf is designed to minimize network load and can serialize and deserialize data quickly.
Required, optional and default values
In the proto3 syntax, all fields are considered optional, and there is no required keyword (which was present in proto2). Proto3 fields are never null but are initialized with default values. Here are the default values for popular types in proto3:
- 0 for numeric fields
- false for booleans
- “” (empty string) for strings
- null for nested message fields (but protobuf provides default instances for them, ensuring null-safe behavior in Java).
Proto3 enforces compatibility even when fields are removed or renamed, as long as you follow best practices such as not reusing field tags.
Proto files backward compatibility
The major advantage of protobuf is backward compatibility. If you update a service’s protobuf definition, gRPC will handle changes safely. You can add new fields or rename existing ones (as long as the field tag remains the same) without breaking backward compatibility. Here’s an updated example of a Car message:
proto
message Car {
int32 id = 1;
string name = 2;
string description = 3;
Color color = 4;
}
enum Color {
UNSPECIFIED = 0;
BLACK = 1;
PINK = 2;
RED = 3;
}
This change does not break backward compatibility because the field tags (1, 2, 3, 5) remain the same. However, you should avoid reusing or renumbering these tags.
Using gRPC in Java
gRPC code generation in Java remains straightforward with the introduction of new tools and optimizations. Key features of generated Java code include:
- Immutability and Null-Safety: The generated classes are immutable and null-safe. You can chain getters without worrying about NullPointerException. For example:
Car reply = Car.newBuilder().setName("Tesla").build();
Engine engine = reply.getEngine(); // Will return a default Engine if not set
int engineCapacity = engine.getCapacity(); // No NullPointerException, uses default value (0)
This feature is especially useful for avoiding common issues in multi-threaded environments, as protobuf-generated code is thread-safe.
- Nested Messages Handling: If a nested message is not set, it will be initialized with default values rather than null, making it easy to avoid NullPointerExceptions. You can also check whether a message field is set with hasX() methods:
if(response.hasEngine()) {
System.out.println("This car has an Engine");
}
Field Masks (Updated for Patch Operations)
FieldMasks continue to play an essential role in partial updates, enabling selective updating of fields, much like the PATCH method in REST. However, with the latest gRPC updates, FieldMask support has been streamlined with better utilities for working with field subsets.
A new tool, FieldMaskUtil, simplifies working with FieldMasks, ensuring that only the specified fields are modified. When using FieldMask, you specify a set of field names (not tags), which prevents issues when fields are renamed or added.
New Features in gRPC (2024)
Recent gRPC improvements include:
- gRPC-Web: gRPC now fully supports Web clients, making it easier to build web applications using gRPC with automatic fallbacks to REST if needed.
- gRPC with Spring Boot: Spring Boot now has official support for gRPC, enabling integration with the Spring ecosystem and making gRPC a first-class citizen in Spring-based microservices.
- Security Enhancements: gRPC has enhanced its security capabilities, including better support for mTLS (mutual TLS) and more flexible authentication/authorization patterns.
Conclusion
gRPC in Java continues to evolve, with improvements in performance, usability, and ecosystem integration. Key features such as contract-first development, protocol buffers, backward compatibility, and null-safety help developers build robust and efficient distributed systems. Whether you are building microservices, real-time communication systems, or working with web clients, gRPC remains a powerful choice for modern APIs.
For more advanced use cases, such as using gRPC with Spring Boot or implementing complex security mechanisms, be sure to refer to the updated documentation and community resources.
Good luck on your coding journey!
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us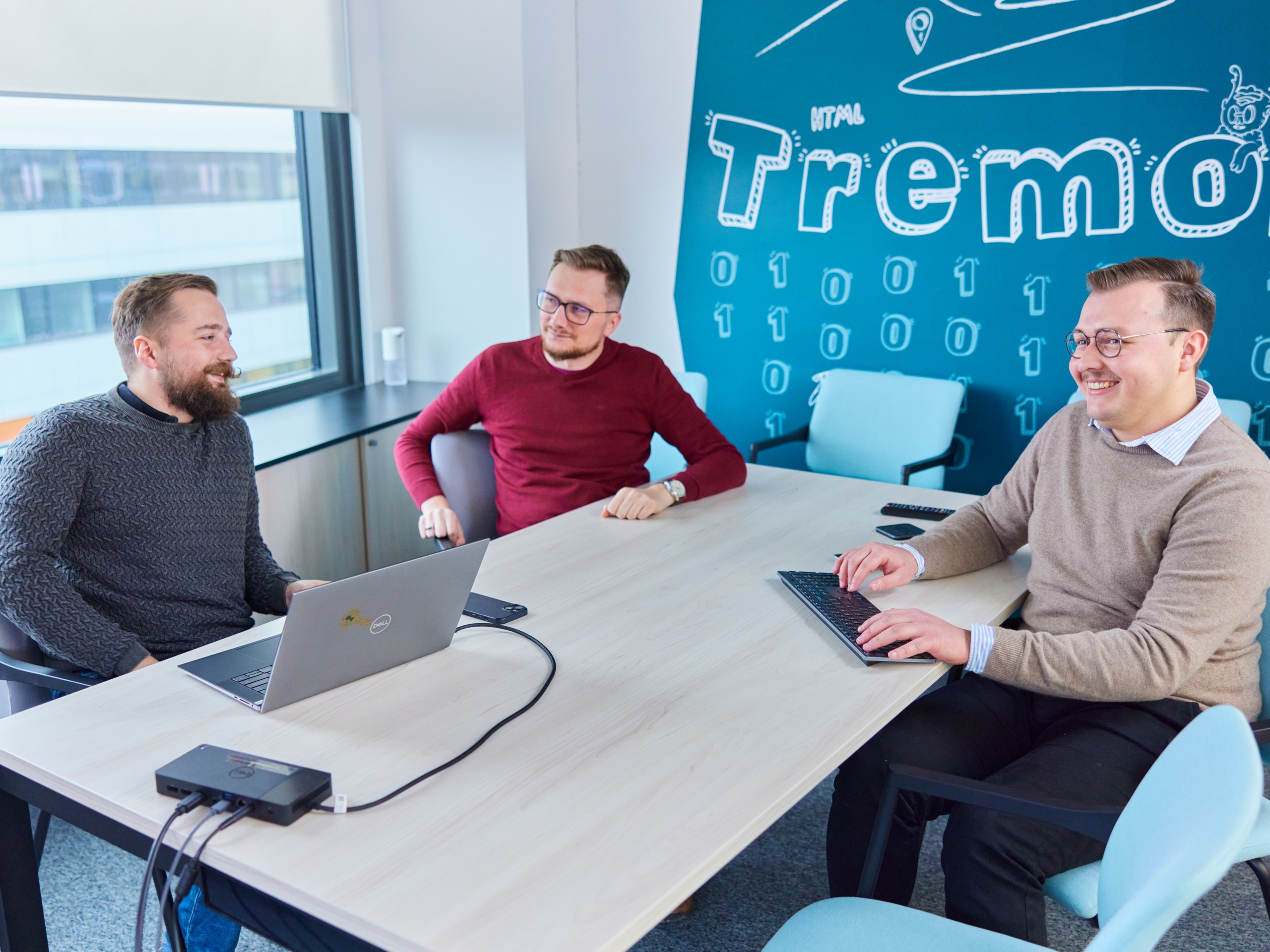
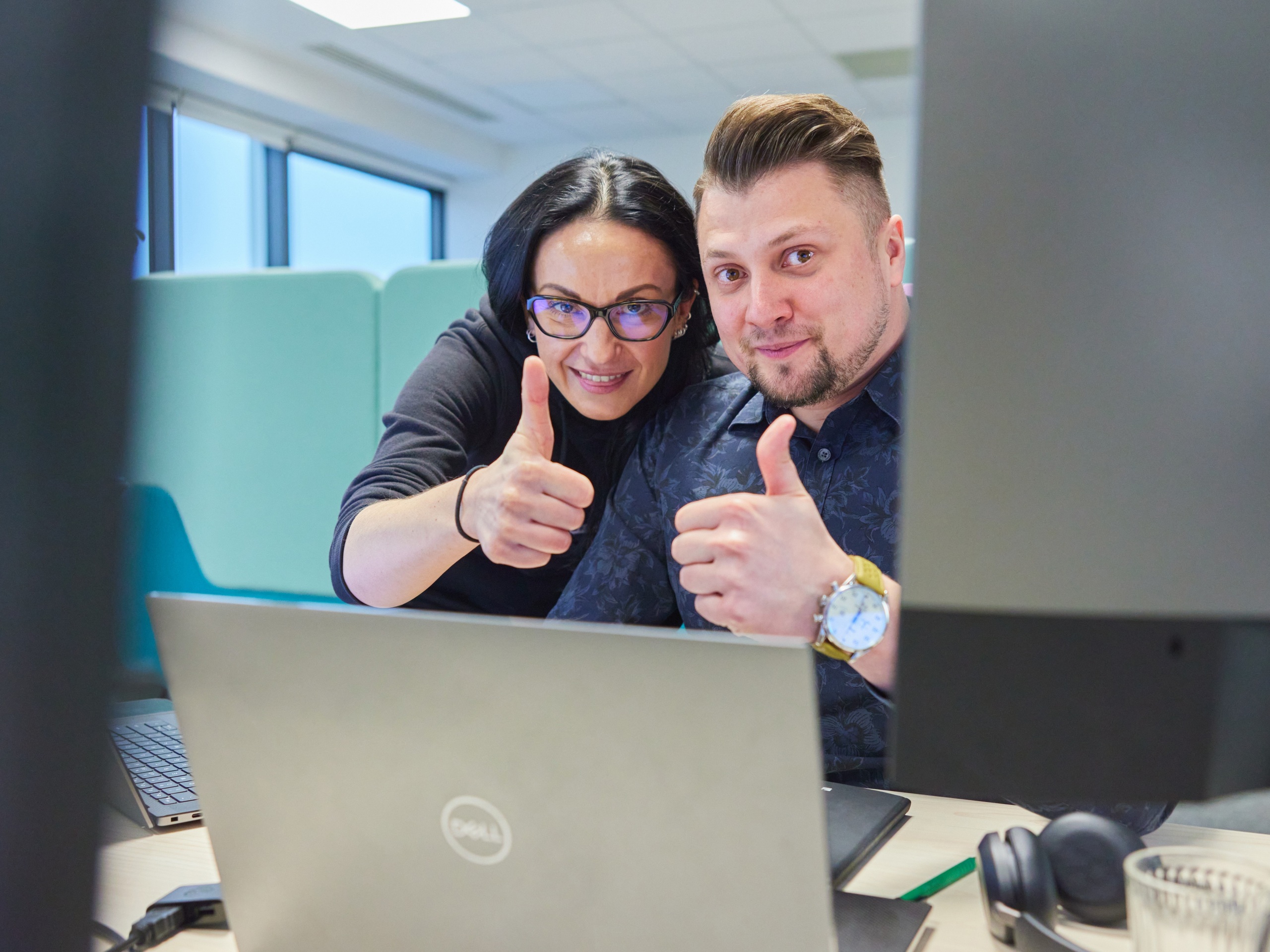
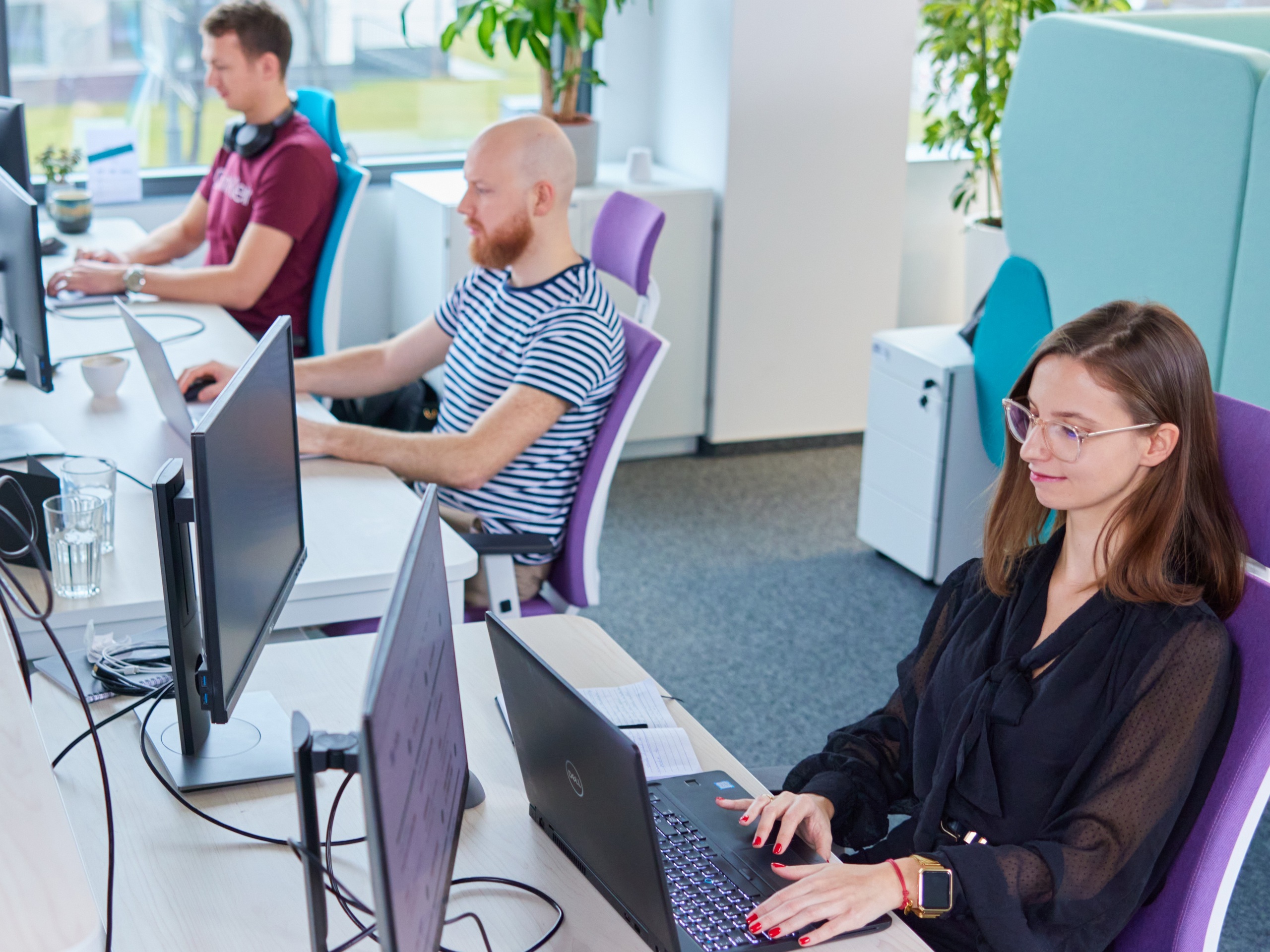
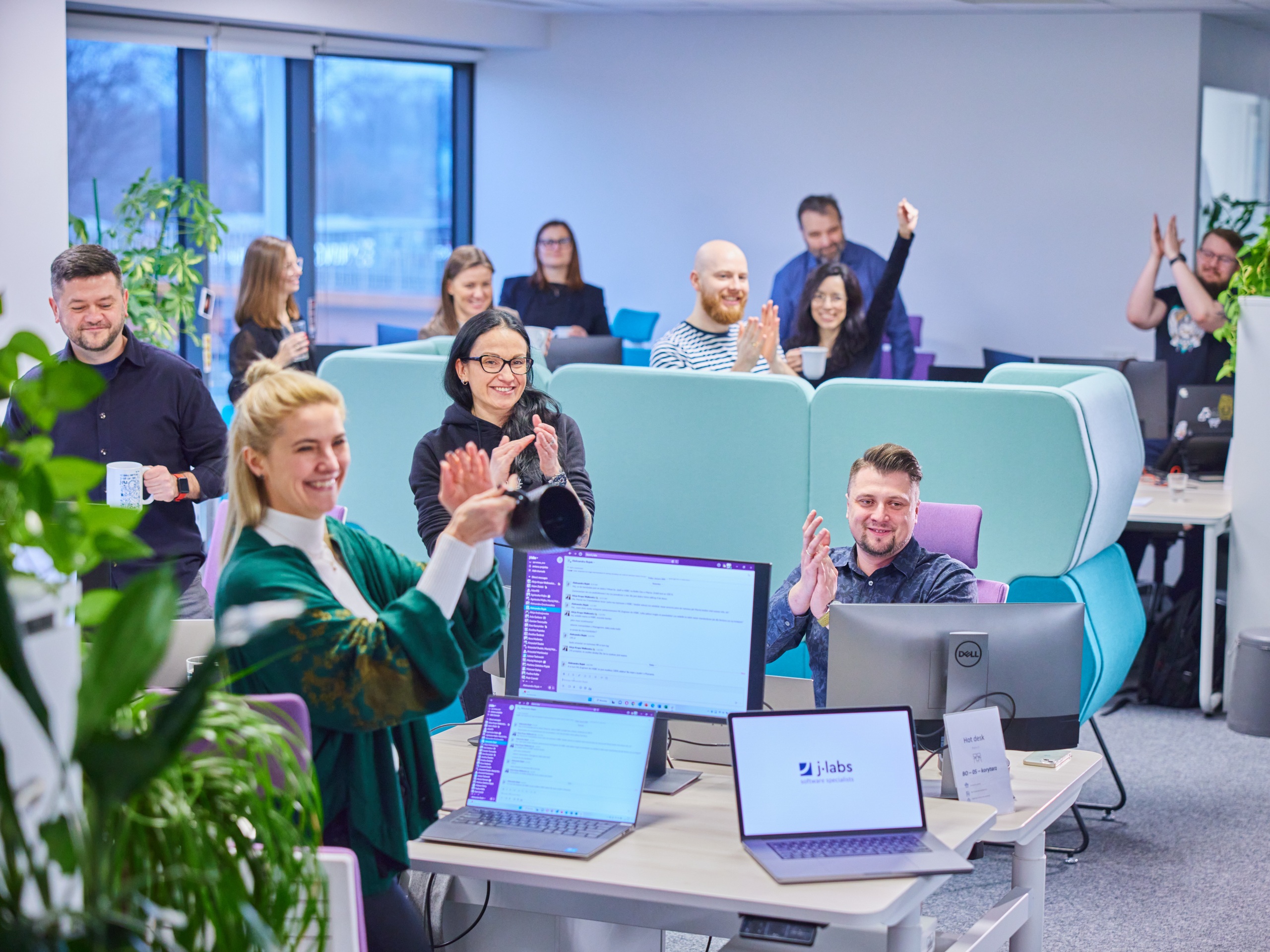