Java Concurrency: Synchronization and Multithreading
Introduction
In the ever-evolving world of software development, Java has emerged as a powerhouse, enabling developers to create robust and efficient applications. A significant aspect of Java’s prowess lies in its ability to handle concurrent execution through multithreading. In this comprehensive article, we embark on a deep dive into Java concurrency, exploring the intricacies of synchronization, multithreading, and the art of harmonizing parallel execution. We’ll uncover the challenges posed by concurrent programming, the mechanisms to tackle them, and provide practical code examples to illustrate these concepts.
Understanding Concurrency and Multithreading
Concurrency, in essence, is the ability of a system to execute multiple tasks simultaneously. Multithreading is a technique that enables a single process to have multiple threads, each executing independently while sharing the same resources. While this concurrent execution brings efficiency, it introduces complexities due to the potential for conflicts and data races.
The Need for Synchronization
When multiple threads access shared resources simultaneously, issues like race conditions and data inconsistencies arise. Java provides synchronization mechanisms to orchestrate thread interactions, ensuring proper coordination and data integrity.
Basic built-in Support for Multi-threaded Programming in Java
Java provides built-in support for multi-threaded programming through its java.lang.Thread
class and the java.util.concurrent
package. This support allows you to create and manage multiple threads of execution within a single Java application, enabling concurrent execution of tasks and improving application performance by utilizing available processor cores. Here are some basic features and classes related to multi-threaded programming in Java:
java.lang.Thread
The Thread class is at the core of Java’s multi-threading support. It represents a single thread of execution within a Java program. You can create a new thread by either extending the Thread class and overriding its run() method or by passing a Runnable object to a Thread constructor.
class ThreadExample extends Thread {
@Override
public void run() {
System.out.println("We are in new Thread!");
}
}
class ThreadTest {
public static void main(String[] args) {
Thread thread = new ThreadExample();
thread.start();
}
}
output:
We are in new Thread!
We are in another Thread!
Runnable
Interface
The Runnable interface represents a task that can be executed concurrently. It has a single method run() that you need to implement to define the task’s behavior. By implementing the Runnable interface, you can decouple the task’s logic from the thread management logic.
class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("We are in Thread " + Thread.currentThread().getName());
}
}
class ThreadTest {
public static void main(String[] args) {
Runnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start();
// starting new Thread on the fly
new Thread(new MyRunnable()).start();
}
}
output:
We are in Thread Thread-0
We are in Thread Thread-1
In both approaches, the run() method defines the code that will be executed by the new thread. The start() method is used to begin the execution of the thread. When you call the start() method, the new thread’s run() method is executed concurrently with the main thread or any other threads that might be running.
It’s important to note that creating and starting a new thread has some overhead, so using thread pooling (through classes like ExecutorService) is often recommended for managing multiple threads efficiently. We’ll delve deeper into more advanced multithreading techniques in another article.
Thread States
In Java, threads can exist in various states during their lifecycle. These states represent the different stages a thread goes through, from its creation to its termination. Understanding thread states is essential for effective multi-threaded programming. Here are the different thread states in Java:
- New State
A thread is in the “new” state when it’s created but not yet started. In this state, the thread’s start() method has not been invoked.
- Runnable State
Once the start() method is called on a thread, it moves to the “runnable” state. In this state, the thread is eligible to be executed by the CPU, but it may not be actively running at every instant due to the CPU scheduler.
- Running State
When the CPU scheduler selects a thread from the “runnable” pool to execute, the thread enters the “running” state. It’s actively executing its code.
- Blocked (or Waiting) State
A thread can move to the “blocked” state if it’s waiting for a particular condition to be satisfied. This can occur when the thread is waiting for I/O operations, synchronization locks, or other events. Once the condition is met, the thread can move back to the “runnable” state.
- Timed Waiting State
A thread can enter the “timed waiting” state if it’s waiting for a specific period of time. This state is often encountered when using methods like Thread.sleep() or waiting for a thread to complete using methods like join().
- Terminated (Dead) State
A thread enters the “terminated” state when its run() method completes or when an unhandled exception occurs within the thread. Once a thread is terminated, it cannot be restarted or resumed.
It’s important to note that threads can transition between these states based on factors like CPU scheduling, synchronization, and I/O operations. Proper synchronization mechanisms and inter-thread communication techniques should be used to manage these state transitions effectively and prevent issues like deadlocks or race conditions.
Monitoring and understanding thread states are crucial for debugging and optimizing multi-threaded applications. Java provides tools like thread dumps and profilers that can help you identify the states of threads and potential issues in your application.
Thread Synchronization
Thread synchronization is a fundamental concept in multi-threaded programming that ensures that multiple threads can access shared resources or perform critical sections of code in an orderly and controlled manner. It prevents race conditions, where the behavior of a program depends on the relative timing of events in multiple threads.
In Java, synchronization is primarily achieved using the synchronized keyword, along with methods like wait(), notify(), and notifyAll(), which are provided by the Object class. These mechanisms enable threads to coordinate their activities and avoid issues like data corruption and deadlocks.
Here’s an explanation of each of these synchronization mechanisms along with examples:
- The
synchronized
Keyword
The synchronized keyword is used to create a critical section, also known as a synchronized block or method. Only one thread can execute the synchronized block at a time, ensuring that shared resources are accessed in a controlled manner.
An example using synchronized block:
public void synchronizedMethod() {
// Only one thread can enter this block at a time
synchronized (this) {
// Critical section of code
}
}
- wait(), notify(), and notifyAll() Methods
These methods provide a way for threads to communicate and coordinate their actions. They should be used within synchronized blocks.
- wait(): Causes the current thread to release the lock and wait until another thread invokes notify() or notifyAll() on the same object.
- notify(): Wakes up a single waiting thread, if any, which was blocked on the same object’s wait() method call.
- notifyAll(): Wakes up all waiting threads that were blocked on the same object’s wait() method call.
An example using wait() and notify():
class SharedResource {
private boolean flag = false;
synchronized void produce() {
while (flag) {
try {
wait(); // Releases the lock and waits
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Produce data
flag = true;
notify(); // Notifies a waiting thread
}
synchronized void consume() {
while (!flag) {
try {
wait(); // Releases the lock and waits
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Consume data
flag = false;
notify(); // Notifies a waiting thread
}
}
In the example above, two threads interact with a shared resource using synchronization and inter-thread communication. The produce() method is executed by one thread and the consume() method by another thread. The wait() and notify() mechanisms ensure that the threads take turns executing and avoid overconsumption or overproduction.
Thread synchronization is essential for maintaining data consistency, avoiding race conditions, and ensuring the correct execution of multi-threaded programs. However, improper use of synchronization can lead to deadlocks or inefficient performance, so it’s important to design synchronization carefully based on the requirements of your application.
Summary
We get a foundational understanding of multithreading’s significance and the pivotal role synchronization plays in crafting reliable, responsive, and efficient Java applications. Now we are equipped with essential insights to embark on the journey of mastering concurrent programming in the Java landscape.
Reference
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us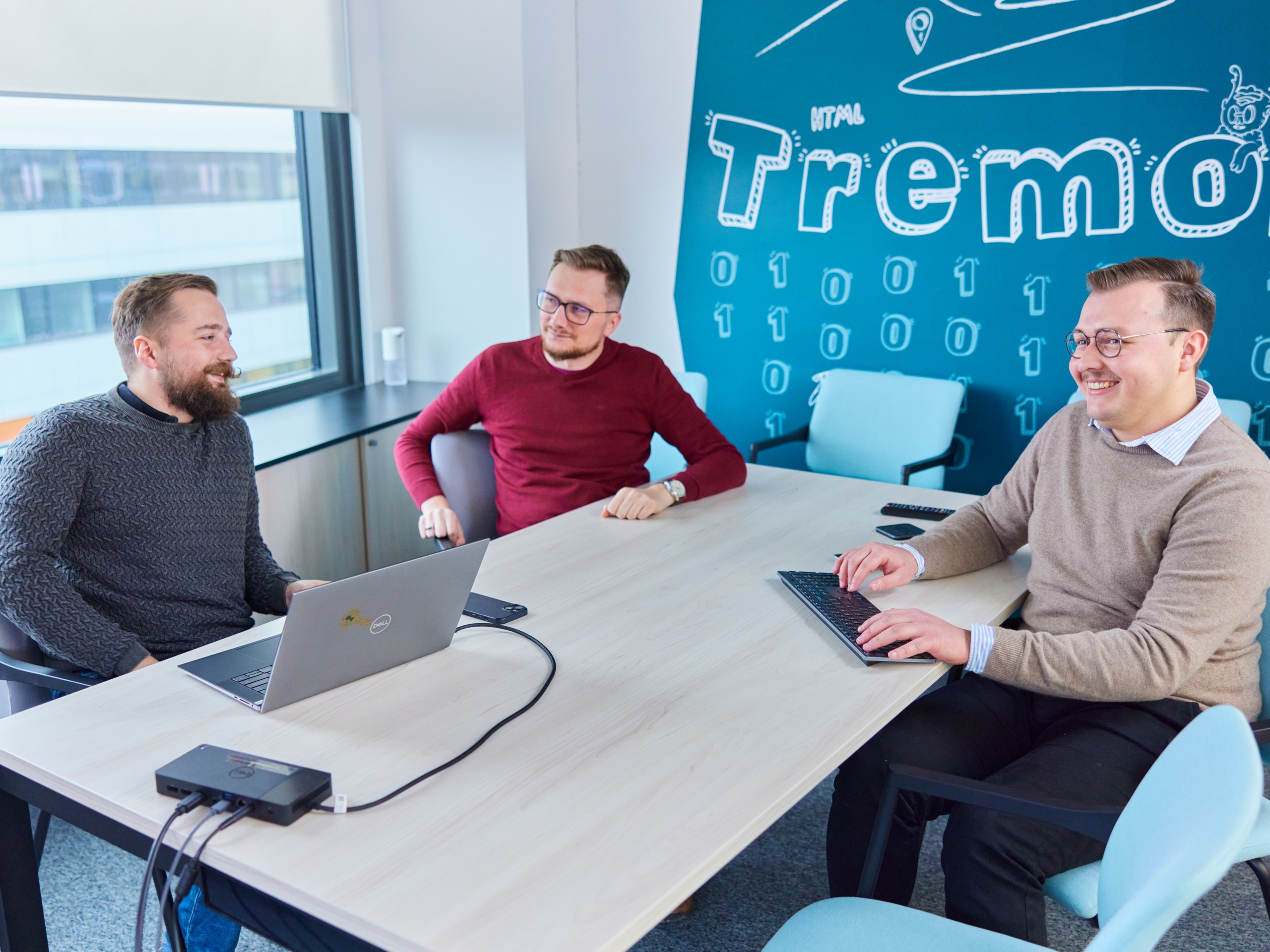
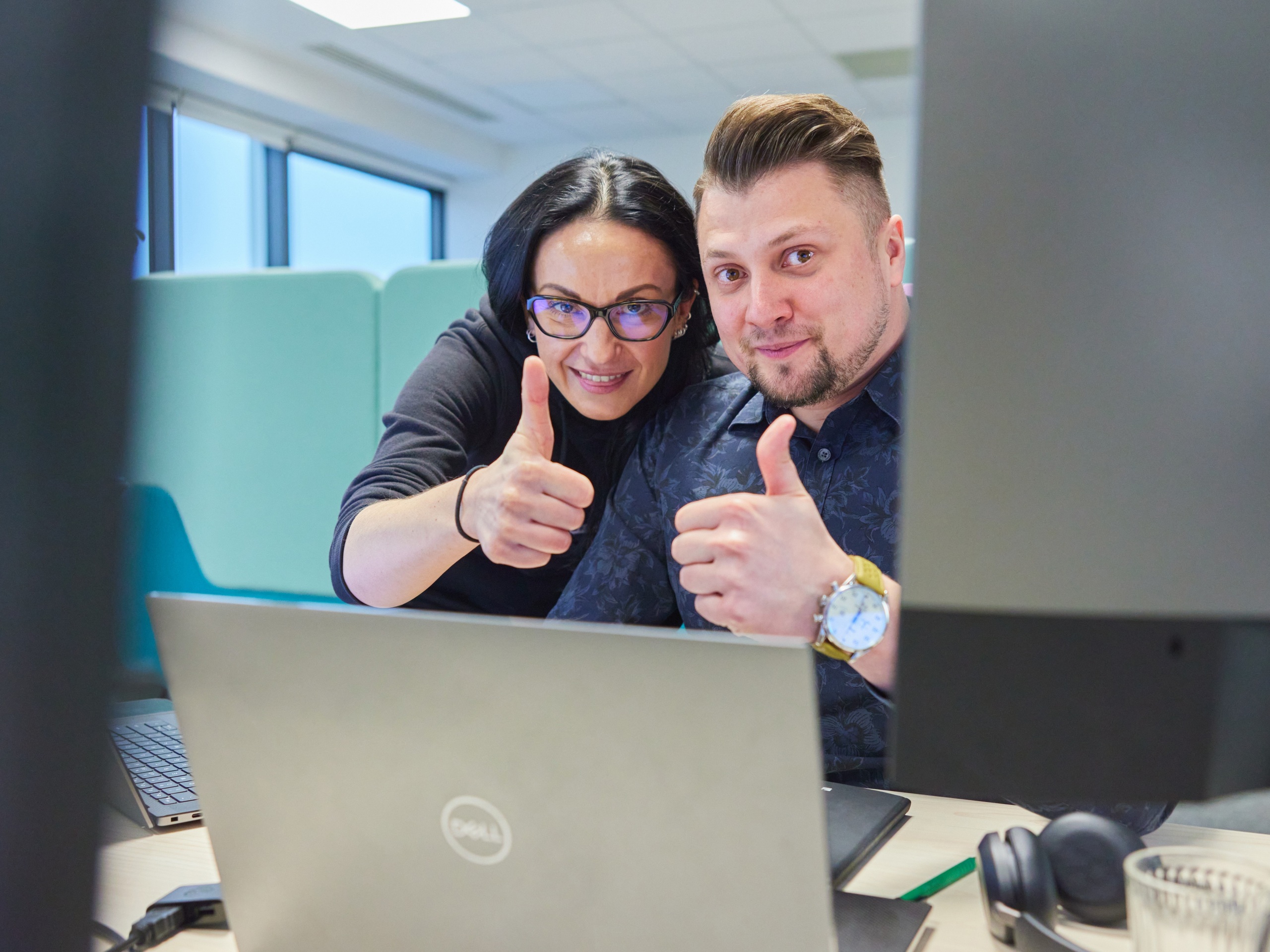
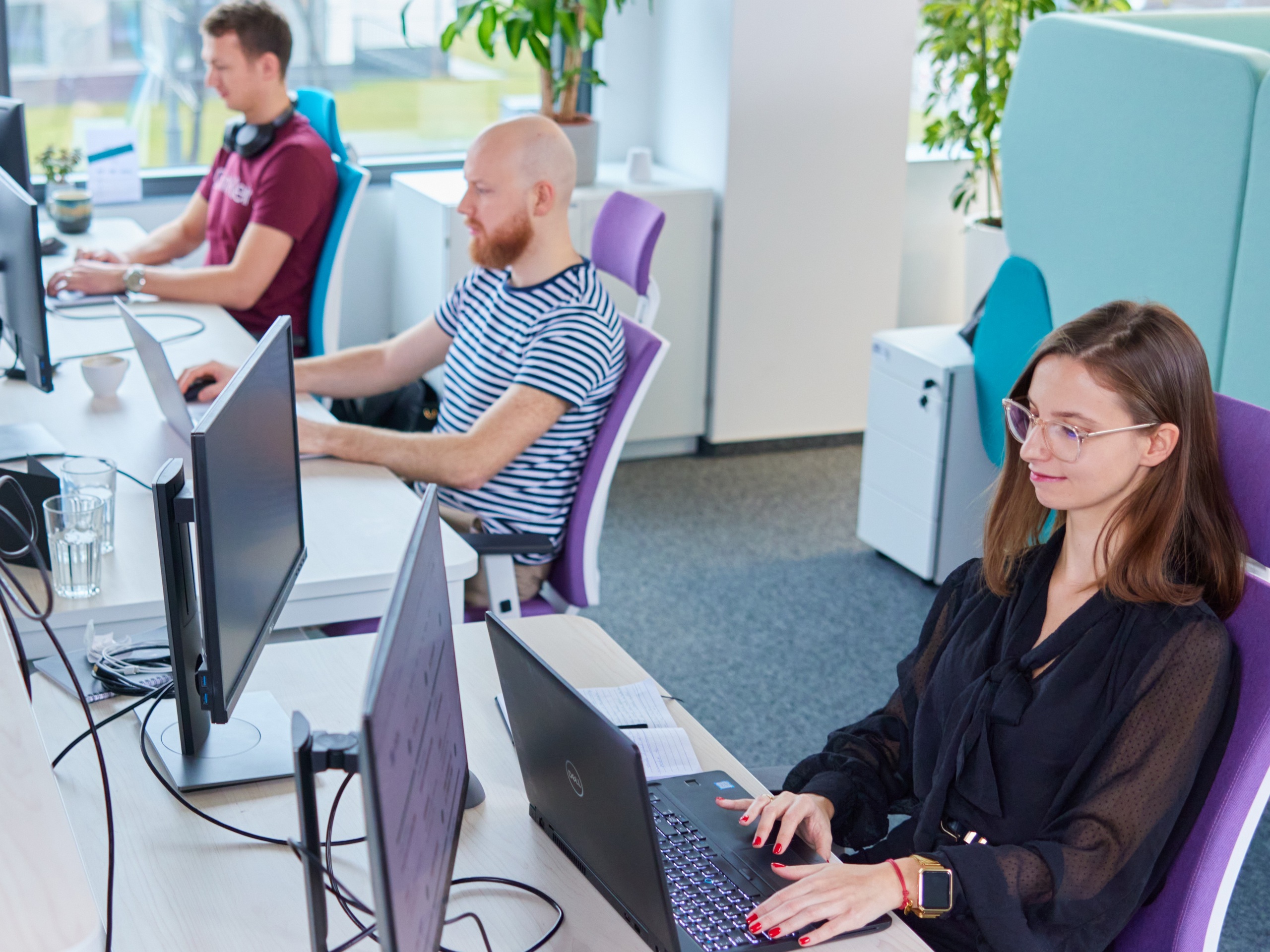
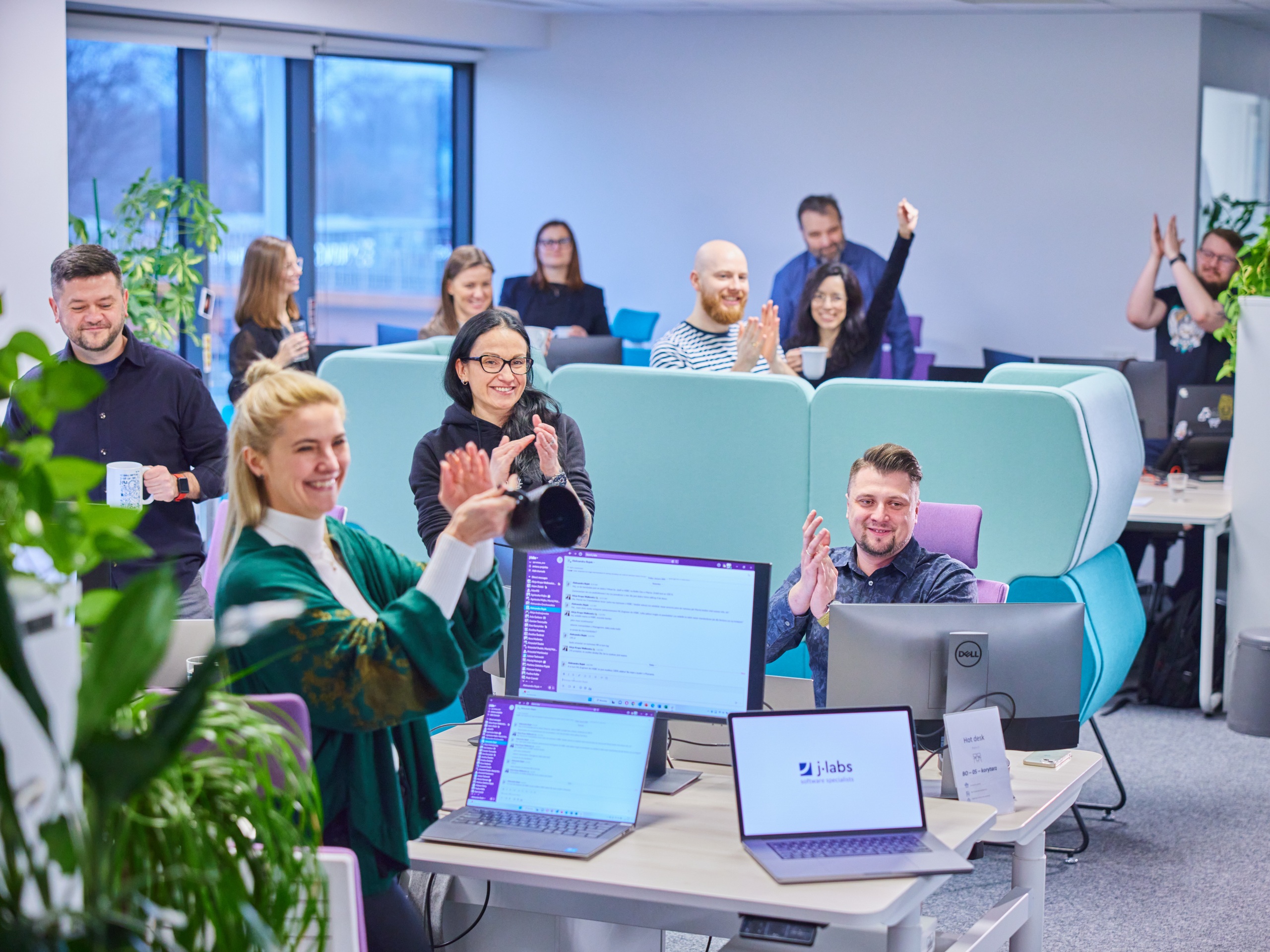