Java Exception Handling: Strategies and Best Practices
Exception handling is a critical aspect of Java programming that allows developers to manage errors and unexpected situations gracefully. Proper exception handling enhances the reliability and maintainability of the code. In this article, we will delve into best practices and strategies for effective exception handling in Java.
Introduction to Java Exception Handling
Exception handling is the process of dealing with runtime errors, ensuring that applications can gracefully recover from unexpected scenarios. Java’s exception handling mechanism involves try-catch blocks, where the code that might throw an exception is enclosed in a try block, and a possible exception handling code is placed in corresponding catch blocks.
Common Types of Java’s Exceptions
In Java, exceptions are categorized into three main types based on their origin and behavior: checked exceptions, unchecked exceptions, and errors. This categorization helps developers understand the nature of exceptions and how they should be handled in the code.
The exception hierarchy in Java is organized into a class hierarchy that inherits from the Throwable class. Here’s a simplified diagram of the exception hierarchy in Java:
┌─────────────────┐
│ Throwable │
└─────────────────┘
/ \
/ \
┌──────┐ ┌─────────┐
│ Error│ │Exception│
└──────┘ └─────────┘
/ \
/ \
┌─────────┐ ┌──────────────────┐
│ Checked │ │ Unchecked │
└─────────┘ │(RuntimeException)│
└──────────────────┘
Here’s a brief explanation of each level of the hierarchy:
- Throwable: This is the root class of the exception hierarchy. Both errors and exceptions inherit from this class. It provides methods like getMessage() and printStackTrace().
- Error: These exceptions are typically thrown by the JVM itself to indicate serious problems that usually cannot be handled by the application code. Examples include OutOfMemoryError and StackOverflowError.
- Exception: These exceptions are more specific and can be either checked or unchecked. Checked exceptions must be caught or declared using the throws clause in the method signature.
- Unchecked (RuntimeException): These are exceptions that often arise due to programming errors or unexpected conditions. They are not required to be caught or declared.
- Checked: These are a subset of exceptions that the compiler requires us to either catch and handle using a try-catch block or declare to be thrown using the throws clause in the method signature. Checked exceptions are typically used to represent exceptional conditions that are recoverable and that the programmer can reasonably anticipate and handle.
This hierarchy helps in categorizing and organizing exceptions based on their nature and behavior. It’s essential to understand this hierarchy when handling exceptions in Java, as it guides you on how exceptions are related and when they need to be caught or declared.
1. Checked Exceptions
Checked exceptions are exceptions that are checked at compile-time, meaning the compiler ensures that these exceptions are either caught using a try-catch block or declared to be thrown using the throws keyword in the method signature. These exceptions usually represent conditions that are beyond the control of the programmer and are typically related to external factors, such as I/O operations or network issues.
Examples of checked exceptions:
- IOException: Raised when there’s an issue with input or output operations, such as reading or writing to files.
- SQLException: Raised for database-related issues.
- ClassNotFoundException: Raised when a class is not found during runtime.
2. Unchecked Exceptions (Runtime Exceptions)
Unchecked exceptions, also known as runtime exceptions, do not need to be declared explicitly in the method signature or caught using a try-catch block. They occur due to programming errors and are often preventable through better coding practices. Unchecked exceptions propagate up the call stack until they are caught or the program terminates.
Examples of unchecked exceptions:
- NullPointerException: Raised when trying to access an object or method on a null reference.
- ArrayIndexOutOfBoundsException: Raised when trying to access an array element with an invalid index.
- IllegalArgumentException: Raised when a method is passed an inappropriate or invalid argument.
- ArithmeticException: Raised when an arithmetic operation is attempted with illegal or undefined values.
3. Errors
Errors represent exceptional conditions that are beyond the control of the application and typically indicate serious problems that may lead to an abnormal termination of the program. Unlike exceptions, errors are not intended to be caught or handled by the application code.
Examples of errors:
- OutOfMemoryError: Raised when the JVM runs out of memory.
- StackOverflowError: Raised when the call stack of a program exceeds its limit.
- NoClassDefFoundError: Raised when the JVM cannot find a class definition.
It’s important to understand these categories of exceptions to write robust and maintainable Java code. Checked exceptions should be handled or declared as appropriate, while unchecked exceptions are usually addressed through better programming practices. Errors are typically not handled by the application code and may require corrective actions at the system level.
Best Practices for Exception Handling
- Use Specific Exception Classes: Handle exceptions at a granular level by catching specific exception classes rather than generic ones.
- Handle Exceptions at the Right Level: Catch exceptions at the level where they can be effectively dealt with.
- Avoid Catching Generic Exceptions: Avoid using catch (Exception e) as it might hide underlying issues.
- Use a Finally Block Sparingly: Use finally only for essential cleanup operations.
- Logging and Reporting Exceptions: Always log exceptions for troubleshooting and debugging purposes.
- Avoid Empty Catch Blocks: Empty catch blocks can lead to silent failures; at least log the exception.
Exception Handling Strategies
- Defensive Programming: Anticipate potential exceptions and handle them proactively.
- Fail Fast: Detect issues as early as possible in the development process.
- Graceful Degradation: Design applications to continue functioning even when exceptions occur.
- Exception Propagation: Allow exceptions to propagate up the call stack if they cannot be handled locally.
public class ExceptionPropagationExample {
public static void main(String[] args) {
try {
method1();
} catch (Exception e) {
System.out.println("Exception caught in main method: " + e);
}
}
static void method1() throws Exception {
method2();
}
static void method2() throws Exception {
method3();
}
static void method3() throws Exception {
// Simulating an exception
int result = 10 / 0; // This will cause an ArithmeticException
// This line won't be reached due to the exception
System.out.println("Result: " + result);
}
}
In this example, we have three methods: method1(), method2(), and method3(). Each method calls the next method in the sequence. In method3(), we intentionally cause an ArithmeticException by dividing an integer by zero.
The exception propagates from method3() to method2(), then from method2() to method1(), and finally from method1() to the main method. The exception is caught in the main method’s try-catch block, and the program doesn’t terminate abnormally.
This example demonstrates how exceptions travel up the call stack until they are caught or the program terminates. It also highlights the importance of handling exceptions appropriately to ensure the robustness of your code.
- Custom Exception Classes: Create custom exception classes for domain-specific errors. Custom exception classes allow you to define your own exception types to represent specific errors or exceptional situations in your application.
// Custom exception class for an insufficient balance scenario
class InsufficientBalanceException extends Exception {
public InsufficientBalanceException(String message) {
super(message);
}
}
// Sample bank account class
class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
public void withdraw(double amount) throws InsufficientBalanceException {
if (amount > balance) {
throw new InsufficientBalanceException("Insufficient balance for withdrawal");
}
balance -= amount;
System.out.println("Withdrawal successful. Remaining balance: " + balance);
}
}
public class CustomExceptionExample {
public static void main(String[] args) {
BankAccount account = new BankAccount(1000.0);
try {
account.withdraw(1500.0);
} catch (InsufficientBalanceException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
In this example, we have defined a custom exception class InsufficientBalanceException that extends the built-in Exception class. This exception is designed to represent situations where there’s not enough balance in a bank account for a withdrawal.
The BankAccount class has a withdraw method that throws the InsufficientBalanceException if the withdrawal amount exceeds the account balance.
In the main method, we create an instance of BankAccount and attempt to withdraw an amount greater than the account balance. Since the withdrawal would lead to an insufficient balance, the InsufficientBalanceException is thrown. We catch this exception and display an error message.
Custom exception classes allow you to encapsulate domain-specific errors and provide meaningful error messages for easier debugging and error handling in your application.
- Try-With-Resources: Use try-with-resources to automatically close resources like files and connections.
Best Practices for Exception Messages in Java
Exception messages play a crucial role in understanding and debugging errors in your code. Well-crafted exception messages can significantly improve the clarity and maintainability of your software. Here are some best practices for creating effective exception messages:
1. Be Descriptive and Clear
Exception messages should clearly convey what went wrong and why. Use plain language to describe the error in a way that is easy for developers to understand.
2. Include Relevant Information
Include context-relevant details in the message. This might include the values of variables, inputs, or conditions that caused the exception. However, avoid exposing sensitive data.
3. Be Consistent
Follow a consistent format for exception messages throughout your codebase. This makes it easier for developers to recognize and handle different types of errors.
4. Use Active Voice
Phrase exception messages in the active voice to clearly indicate the cause of the error. For example, “File not found” is more informative than “File was not found.”
5. Avoid Technical Jargon
While you want to provide meaningful information, avoid using technical jargon that might confuse other developers or stakeholders who read the error messages.
6. Avoid Abbreviations and Acronyms
Spell out terms instead of using abbreviations or acronyms. This ensures that everyone can understand the message, even those who are not familiar with the abbreviations.
7. Provide Potential Solutions
If possible, suggest potential solutions or actions that the developer can take to resolve the issue. This can help in the troubleshooting process.
8. Use Proper Punctuation
Exception messages are part of your application’s user interface, so use proper punctuation, capitalization, and formatting to make them readable and professional.
9. Keep It Short and Precise
Exception messages should be concise and to the point. Avoid lengthy messages that might overwhelm the developer or clutter the log.
10. Localize Messages (if Applicable)
If your software is used in different locales, consider providing localized exception messages to cater to users who speak different languages.
11. Include Stack Trace
When logging exceptions, include the stack trace to provide developers with a detailed view of where the exception occurred in the code. This aids in diagnosing the issue.
12. Avoid Blaming the User
While it’s important to indicate errors, avoid blaming the user directly in the message. Instead, focus on explaining the issue and providing a solution.
Remember that the goal of exception messages is to help developers identify and fix issues quickly. By following these best practices, you can make your error messages more informative, user-friendly, and conducive to effective troubleshooting and debugging.
Exceptions in Functional Programming
In functional programming, the approach to handling exceptions is slightly different from traditional imperative programming. Functional programming emphasizes immutability, pure functions, and avoiding side effects. While exceptions are still used to handle errors, they are typically managed in a way that aligns with the functional programming principles. Here’s how you can handle exceptions in functional programming using Java:
Optional
Instead of throwing exceptions, functional programming often uses option types (like Optional in Java). These constructs allow you to represent success or failure explicitly and safely.
Optional<Integer> divide(int a, int b) {
if (b == 0) {
return Optional.empty(); // Division by zero case
}
return Optional.of(a / b);
}
Function Composition
Functional programming promotes composing functions to transform data. When dealing with exceptions, you can chain functions that handle different error cases.
Optional<Double> calculateRiskFactor(int age, int speed) {
return divide(speed, age)
.map(ratio -> Math.sqrt(ratio))
.filter(risk -> risk < 10);
}
Side-effect free Functions
In functional programming, functions should be side-effect free. This means that functions should not modify the external state. Instead of directly modifying the external state, return updated copies of data.
Exception handling in Java – Summary
Effective exception handling is a cornerstone of robust Java applications. By following best practices and adopting appropriate exception handling strategies, developers can build applications that gracefully handle errors, provide meaningful feedback to users, and maintain a high level of reliability.
In this article, we have discussed essential best practices, strategies, and real-world examples that empower Java developers to master the art of exception handling, enhancing the overall quality of their software projects.
Check also article about weka machine learning
Reference
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us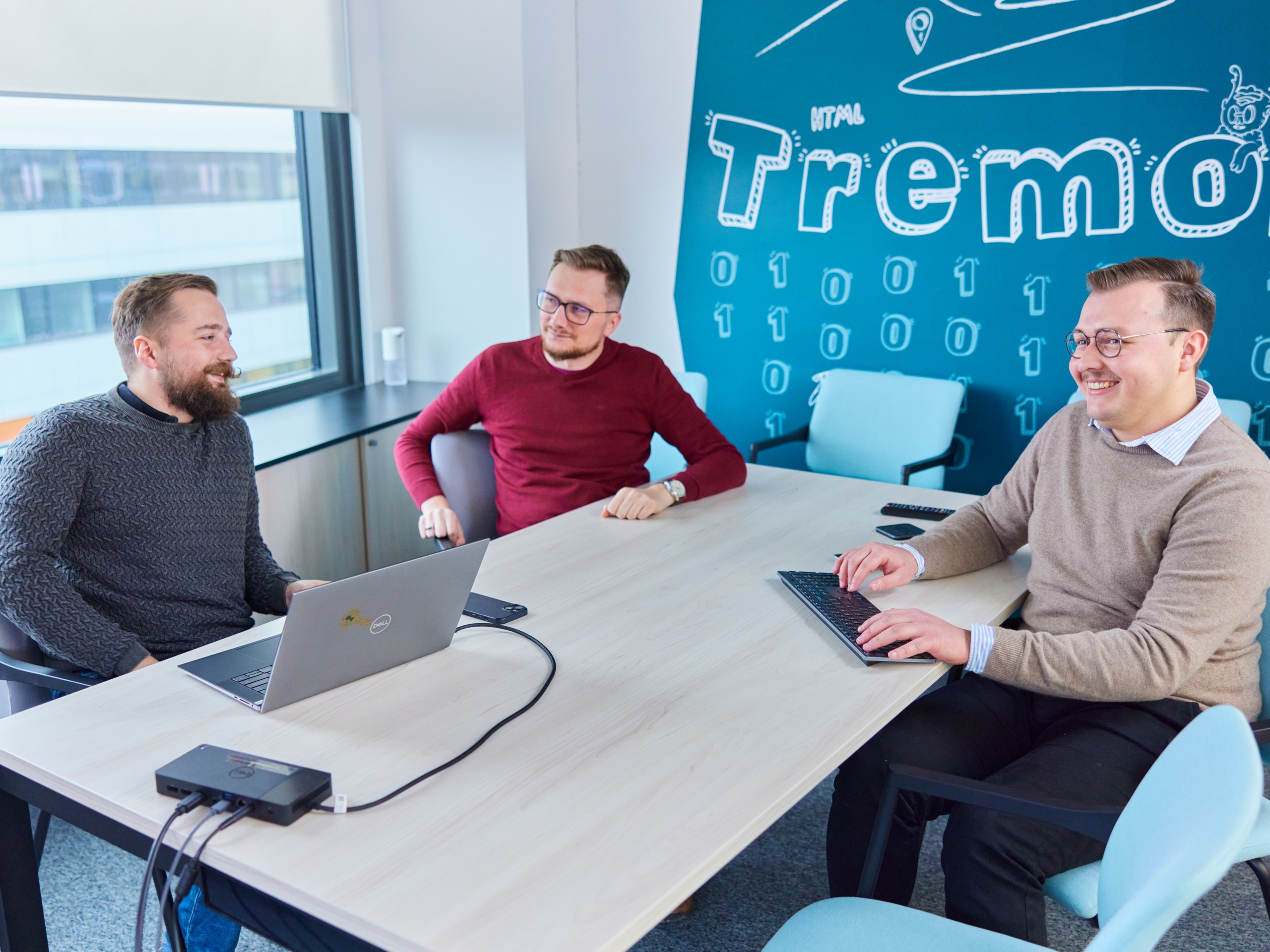
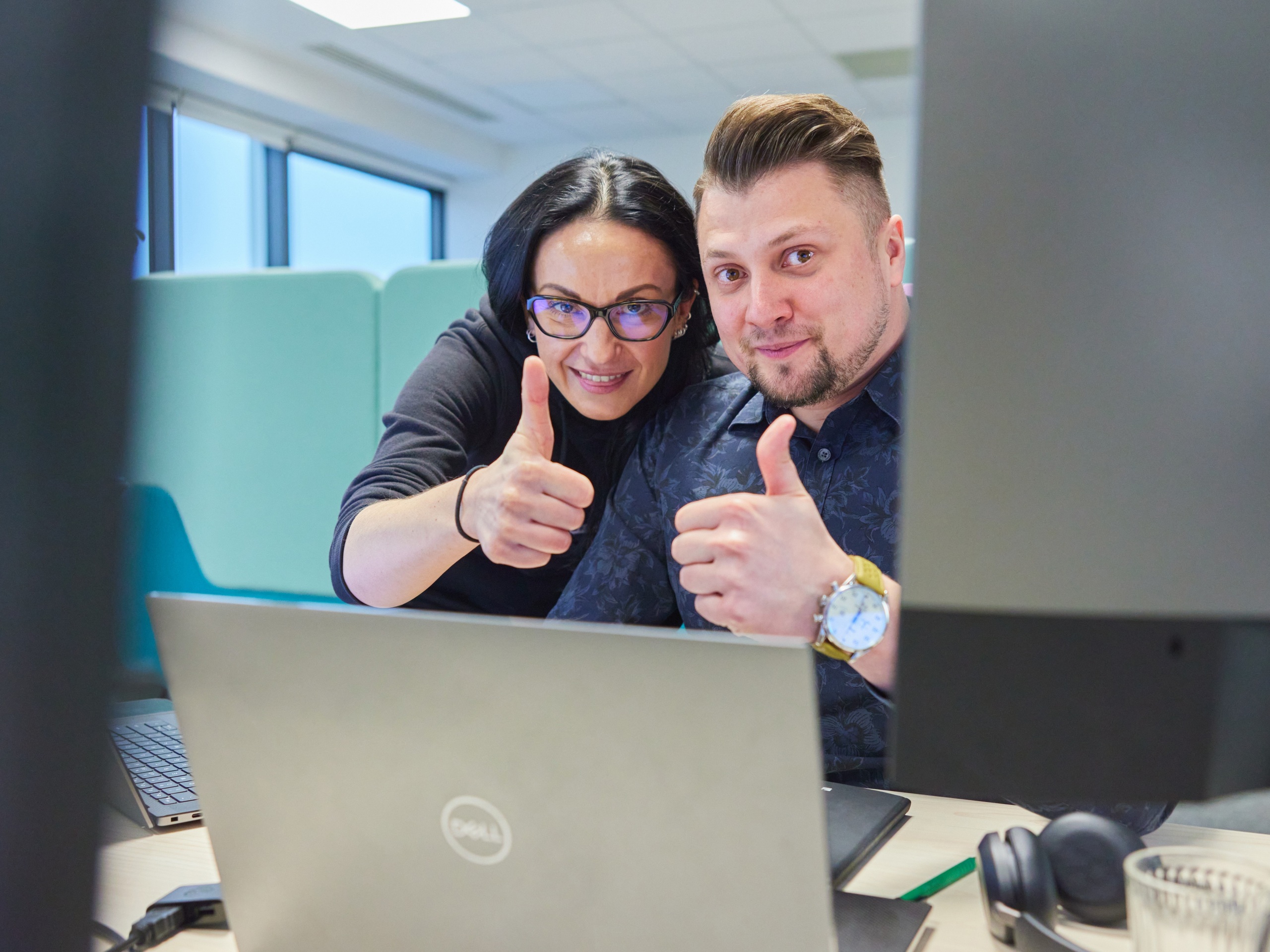
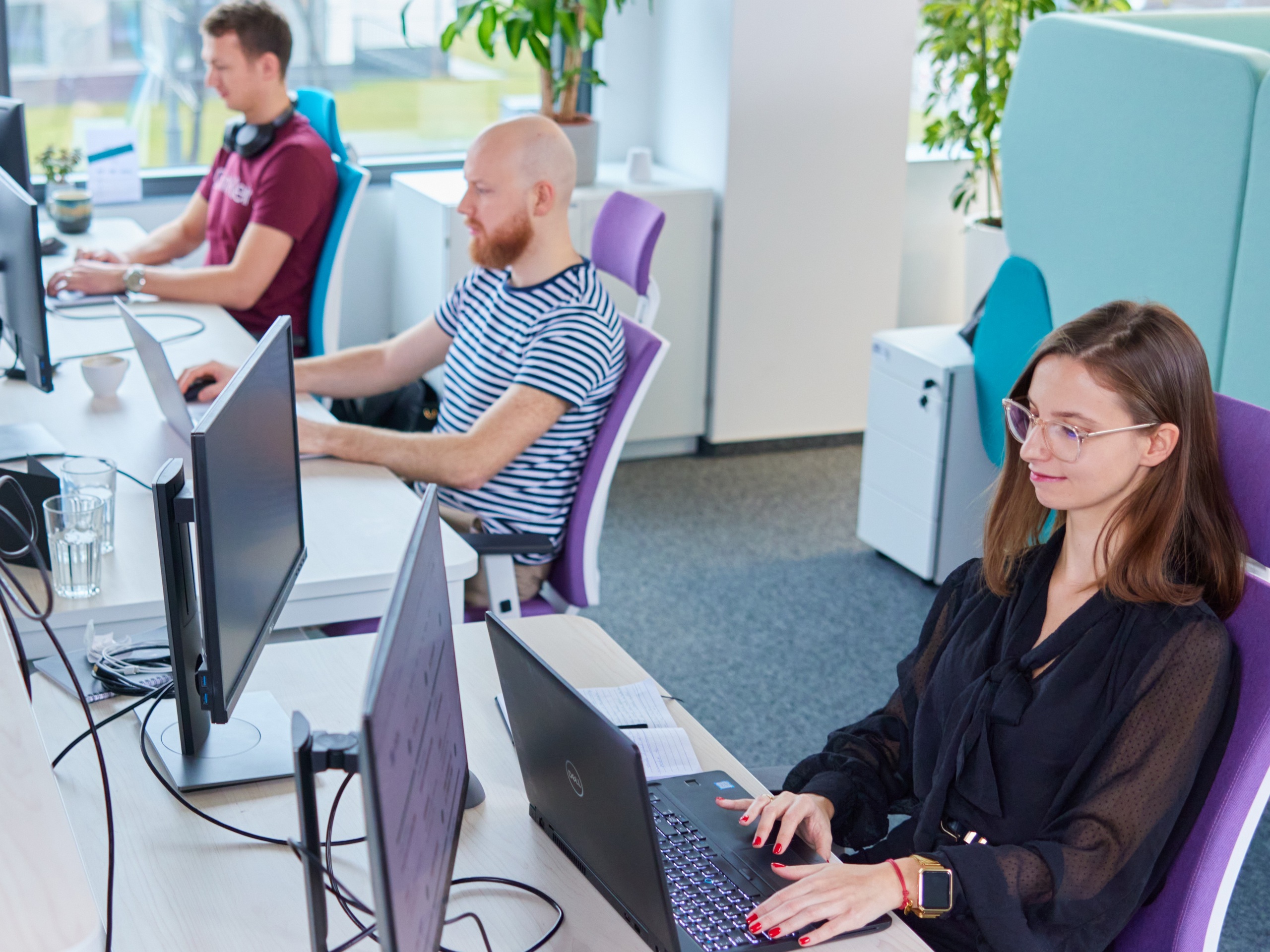
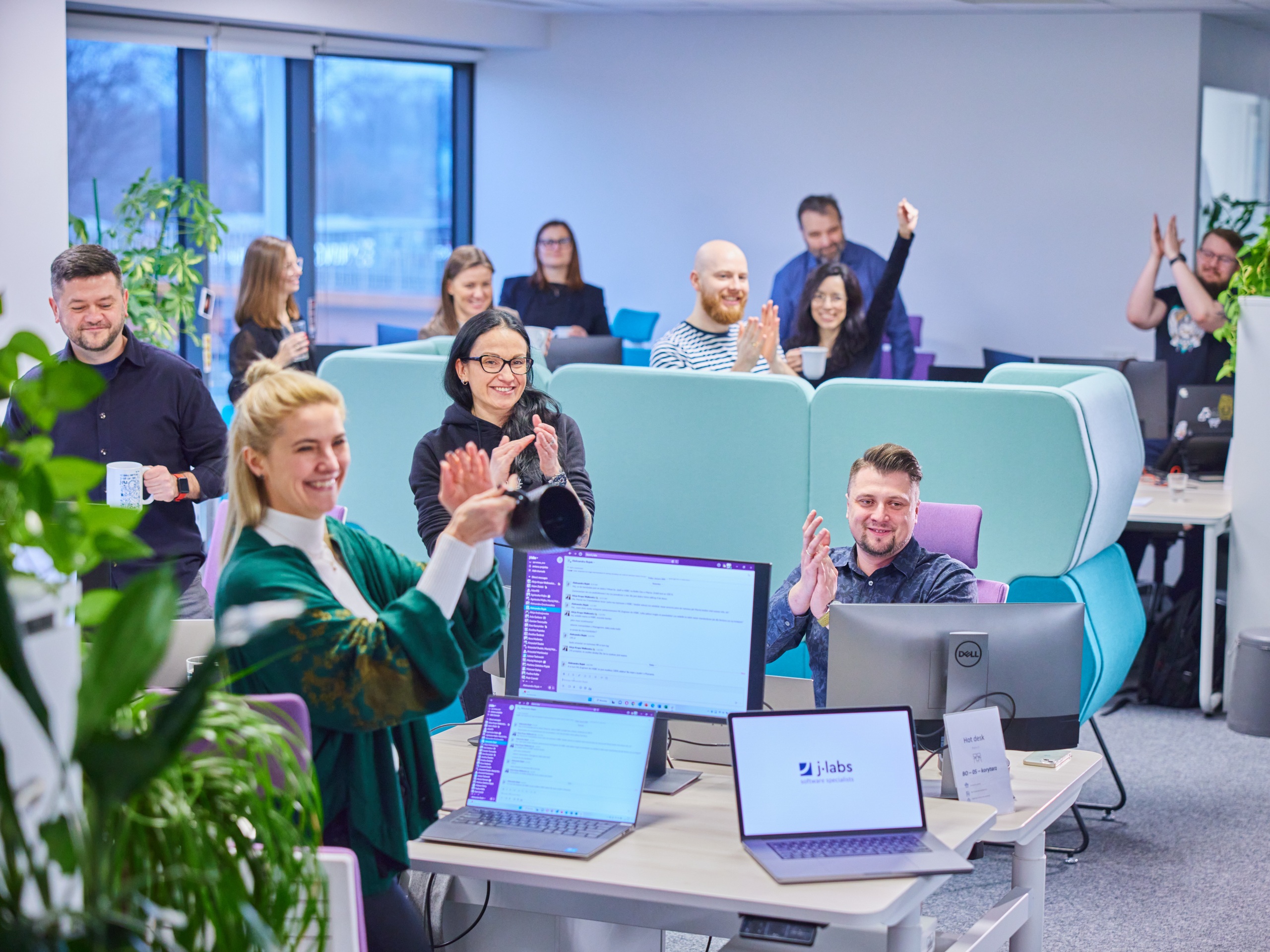