Machine Learning in Java: Getting Started with Weka
Introduction
Machine learning has emerged as a transformative technology, empowering computers to learn from data and make intelligent decisions. Among the plethora of machine learning tools, Weka stands as a powerful and user-friendly framework, offering a seamless gateway into the world of data-driven insights. In this article, we’ll embark on a journey into the basics of machine learning using Weka in the Java programming language. We’ll explore fundamental concepts, data preprocessing, model training, and evaluation, supported by illustrative Java code examples.
Prerequisites
Before diving into Weka, make sure you have the following set up:
- Java Development Kit (JDK) installed
- Weka library downloaded and added to your Java project
Getting Acquainted with Weka
Weka, an open-source machine learning toolkit, simplifies the complexities of machine learning. It provides a wide array of algorithms for classification, regression, clustering, and more. Let’s start by loading a dataset and applying a basic classification model.
import weka.classifiers.bayes.NaiveBayes;
import weka.core.Instances;
import static weka.core.converters.ConverterUtils.DataSource;
public class WekaDemo {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("iris.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Build and evaluate a model
NaiveBayes classifier = new NaiveBayes();
classifier.buildClassifier(dataset);
// Print model summary
System.out.println(classifier);
}
}
Output:
Naive Bayes Classifier
Class
Attribute Iris-setosa Iris-versicolor Iris-virginica
(0.33) (0.33) (0.33)
===============================================================
sepallength
mean 4.9913 5.9379 6.5795
std. dev. 0.355 0.5042 0.6353
weight sum 50 50 50
precision 0.1059 0.1059 0.1059
sepalwidth
mean 3.4015 2.7687 2.9629
std. dev. 0.3925 0.3038 0.3088
weight sum 50 50 50
precision 0.1091 0.1091 0.1091
petallength
mean 1.4694 4.2452 5.5516
std. dev. 0.1782 0.4712 0.5529
weight sum 50 50 50
precision 0.1405 0.1405 0.1405
petalwidth
mean 0.2743 1.3097 2.0343
std. dev. 0.1096 0.1915 0.2646
weight sum 50 50 50
precision 0.1143 0.1143 0.1143
In this example, we load the classic Iris dataset (“iris.arff”) using Weka’s DataSource. We set the class index and build a simple Naive Bayes classifier using the NaiveBayes class.
Naive Bayes is a machine learning algorithm primarily used for classification tasks. It’s particularly useful when you want to classify data into predefined categories or classes based on input features. The algorithm is based on the Bayes’ theorem and makes an assumption of independence between features, which is why it’s called “naive.”
Despite its “naive” assumption, Naive Bayes often performs well on a wide range of classification tasks, especially when dealing with high-dimensional data like text. It’s relatively simple, computationally efficient, and can provide accurate results with relatively small amounts of training data. However, it might not work well in cases where feature dependencies are crucial for accurate classification or when the dataset is significantly imbalanced.
Finally, we print the model summary.
Data Preprocessing
Data preprocessing is crucial for effective machine learning. Weka provides utilities to handle missing values, normalize data, and more.
Data normalization and discretization are two preprocessing techniques commonly used in machine learning to improve the performance of algorithms and the interpretability of data. These techniques are available as filters in the Weka machine learning toolkit.
Data Normalization
Data normalization, also known as feature scaling, is the process of transforming features so that they have a consistent scale. This is crucial when features have different ranges, and some machine learning algorithms, like gradient descent-based ones, are sensitive to feature scales.
Data Discretization
Data discretization involves converting continuous data into discrete values. This is often done to simplify the data, make it more understandable, and reduce noise. Discretization is particularly useful for algorithms that work with categorical data or that perform better when data is grouped into bins.
import weka.core.Instances;
import weka.filters.Filter;
import weka.filters.supervised.attribute.Discretize;
import weka.filters.unsupervised.attribute.Normalize;
import static weka.core.converters.ConverterUtils.DataSource;
public class DataPreprocessing {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("src/main/java/weka/diabetes.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Normalize data
Normalize normalize = new Normalize();
normalize.setInputFormat(dataset);
Instances normalizedDataset = Filter.useFilter(dataset, normalize);
// Discretize data
Discretize discretize = new Discretize();
discretize.setInputFormat(normalizedDataset);
Instances discretizedDataset = Filter.useFilter(normalizedDataset, discretize);
System.out.println(discretizedDataset);
}
}
In this example, we load the “diabetes.arff” dataset and perform data normalization and discretization using Weka’s filters.
Keep in mind that the choice of normalization and discretization methods should depend on the characteristics of your data and the requirements of your machine learning algorithm.
Using these preprocessing techniques can significantly improve the performance and reliability of your machine learning models by reducing the impact of feature scales and enhancing the interpretability of the data.
Model Evaluation
Model evaluation is a critical step in the machine learning process that involves assessing the performance of a trained model on unseen data. Weka provides various tools and techniques for evaluating models. The primary goal of model evaluation is to understand how well the model generalizes to new data and to identify any potential issues or areas for improvement. Let’s explore the process of model evaluation using Weka:
import weka.classifiers.bayes.NaiveBayes;
import weka.classifiers.evaluation.Evaluation;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class ModelEvaluation {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("src/main/java/weka/heart.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Build a model
NaiveBayes classifier = new NaiveBayes();
classifier.buildClassifier(dataset);
// Evaluate model
Evaluation evaluation = new Evaluation(dataset);
evaluation.evaluateModel(classifier, dataset);
System.out.println(evaluation.toSummaryString());
}
}
Output:
Correctly Classified Instances 255 84.1584 %
Incorrectly Classified Instances 48 15.8416 %
Kappa statistic 0.6795
Mean absolute error 0.068
Root mean squared error 0.2182
Relative absolute error 33.9218 %
Root relative squared error 69.2704 %
Total Number of Instances 303
In this example, we load the “heart.arff” dataset, build a Naive Bayes model, and evaluate its performance using Weka’s Evaluation class.
In summary, model evaluation is a crucial step in the machine learning workflow, enabling you to assess how well your model performs on unseen data. Weka provides a variety of tools and methods for evaluating classifiers, regressors, and other models, along with visualizations to aid in result interpretation and model comparison.
Summary
Weka serves as an excellent entry point to the world of machine learning using Java. Its user-friendly interface, diverse algorithms, and comprehensive toolset empower developers to explore, experiment, and gain insights from their data. As you continue your machine learning journey, delve into more advanced techniques, explore diverse datasets, and refine your models to tackle real-world challenges. With Weka in your arsenal, you’re well-equipped to harness the power of machine learning in the Java ecosystem.
Reference
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us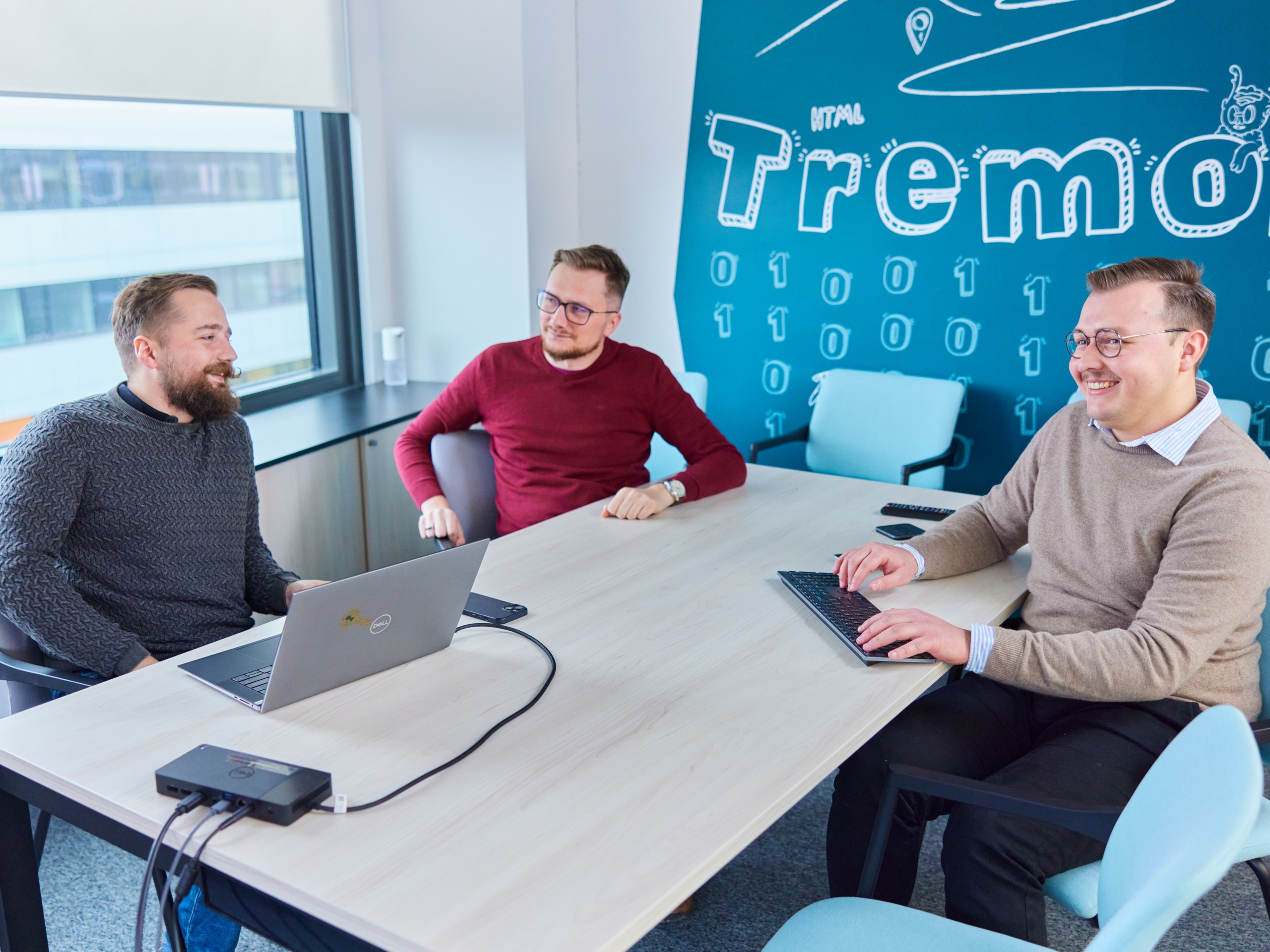
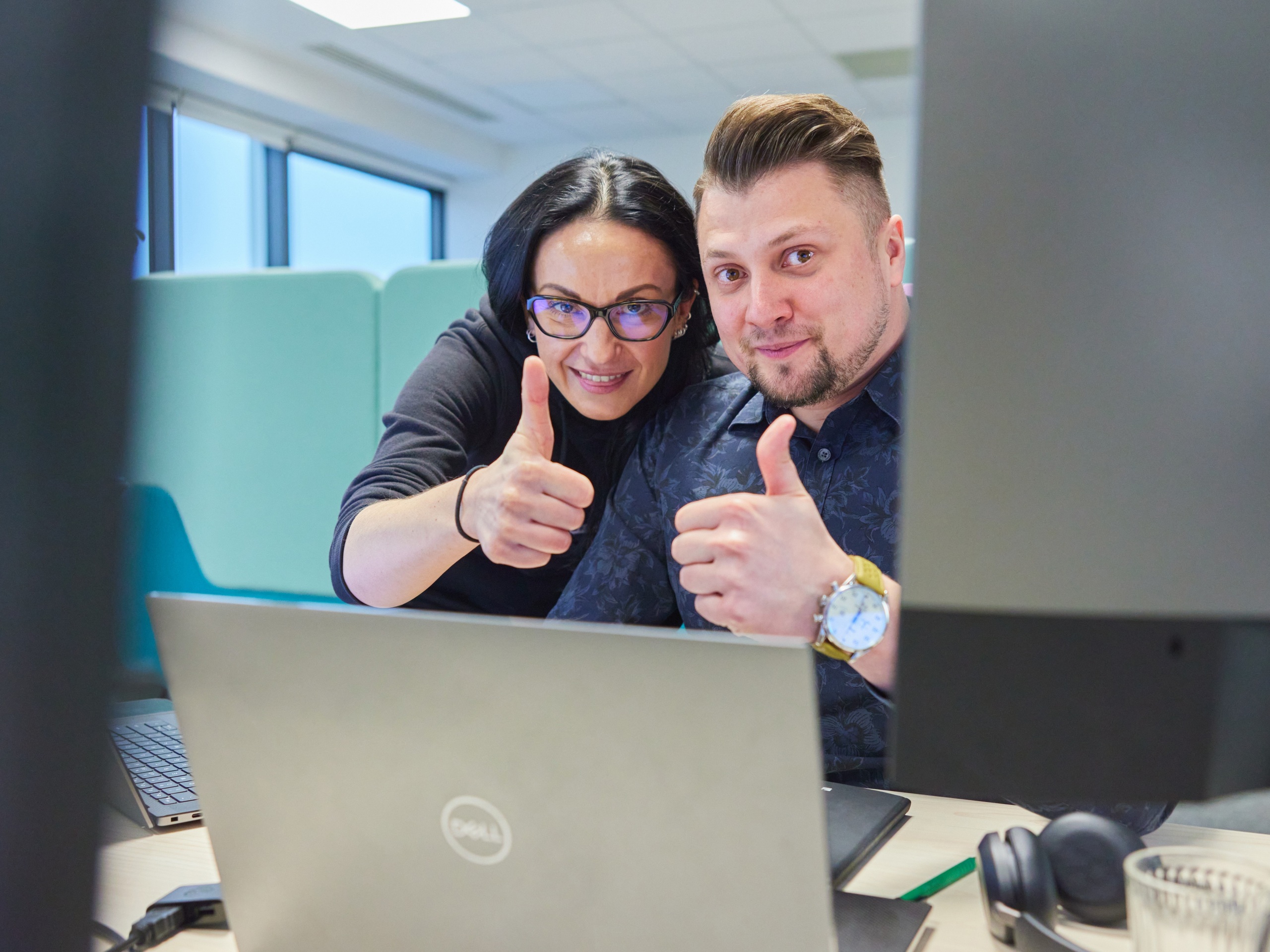
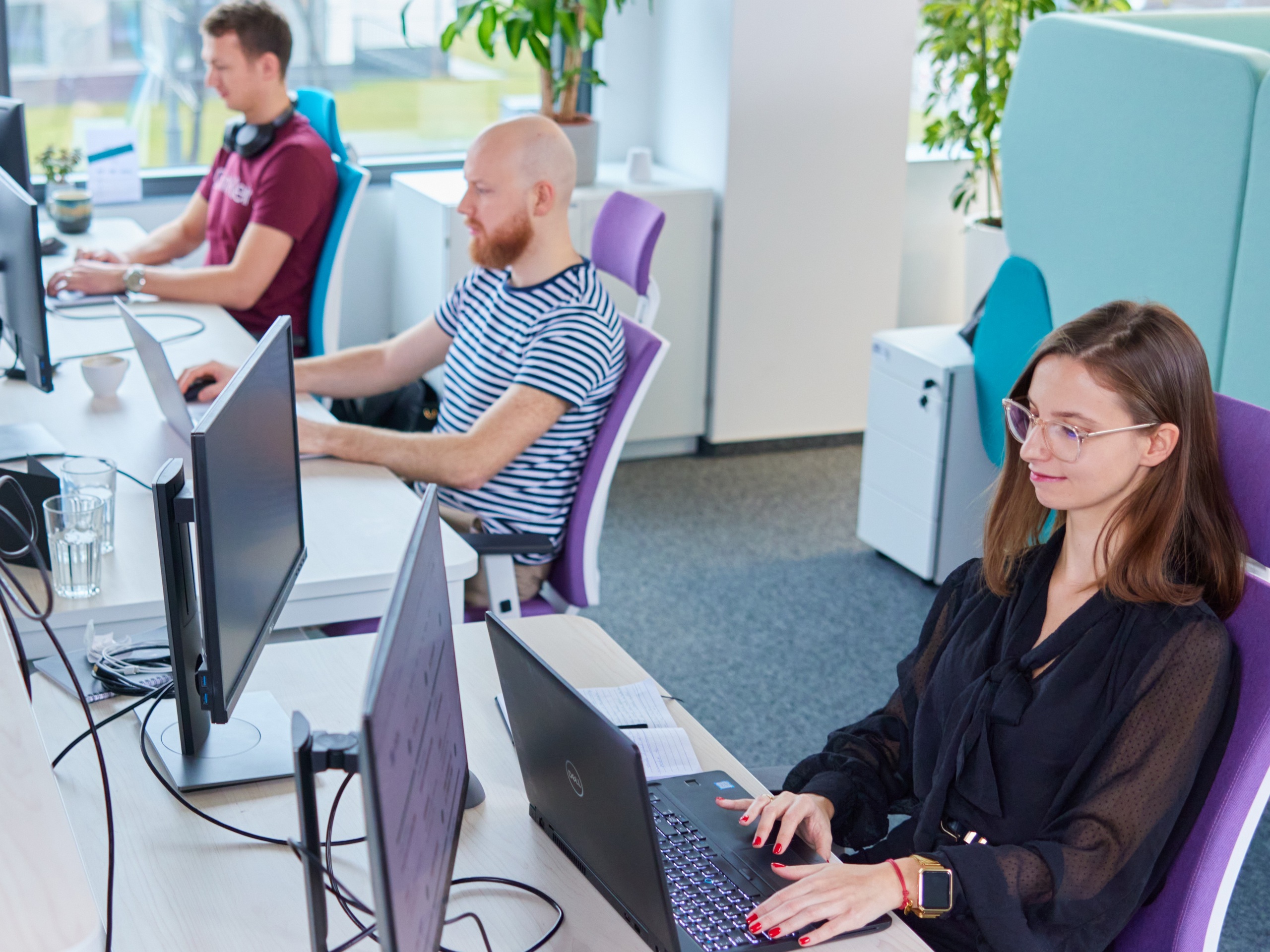
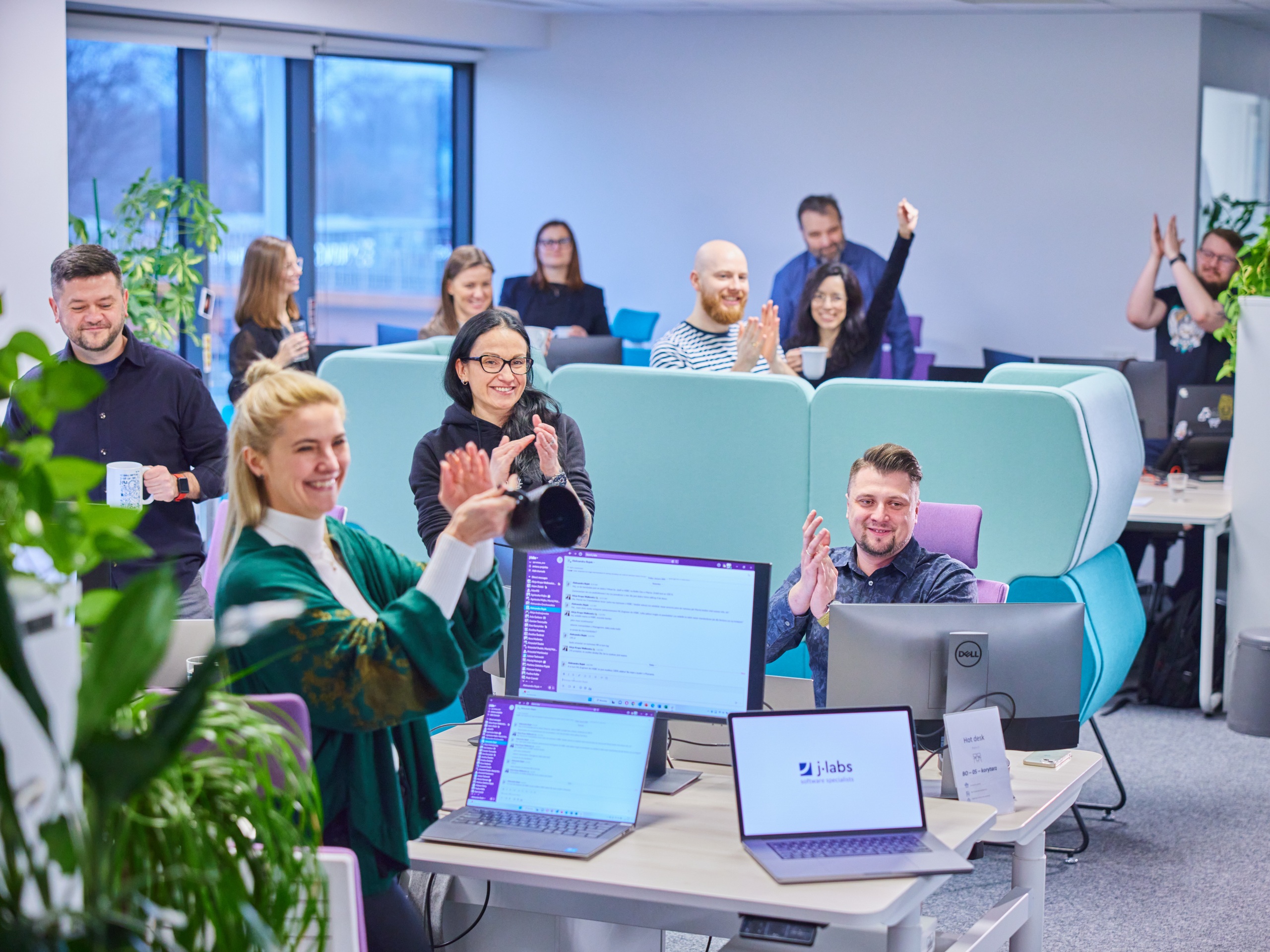