Make Your First Chrome Extension
Introduction
Chrome extensions are a powerful way to enhance the user experience by extending the browser’s capabilities. In this tutorial, we will walk you through the process of creating a simple Chrome extension, including the manifest file, background scripts, and content scripts. We will also provide an example source code to help you get started.
Prerequisites
To follow along with the examples in this article, you should have:
- Basic knowledge of JavaScript, HTML, and CSS.
- The Google Chrome browser installed on your computer.
- A text editor for writing code (e.g., Visual Studio Code, Sublime Text, or Notepad++).
Setting up the extension folder and manifest file
To begin, create a new folder on your computer where you will store all the files related to your Chrome extension. You can name it anything you like, for example, “MyFirstExtension”.
Next, create a new JSON file named “manifest.json” in the “MyFirstExtension” folder. This file contains metadata about your extension and is required for Chrome to recognize and load it. Paste the following code into “manifest.json”:
{
"manifest_version": 3,
"name": "My First Chrome Extension",
"version": "1.0",
"description": "A simple Chrome extension tutorial",
"icons": {
"48": "icon.png"
},
"permissions": ["tabs", "activeTab", "scripting"],
"action": {
"default_icon": "icon.png",
"default_popup": "popup.html"
},
"background": {
"service_worker": "background.js"
},
"content_scripts": [
{
"matches": ["https://*/*"],
"js": ["content.js"]
}
]
}
This manifest file defines basic information about your extension, such as its name, version, and description. It also specifies required permissions, browser action properties, and background and content scripts.
Step 2: Creating an icon and popup
To create a simple icon for your extension, use any image editing tool to create a 48×48 pixel PNG file. Save it as “icon.png” in the “MyFirstExtension” folder.
Next, create a new HTML file named “popup.html” in the “MyFirstExtension” folder. This file will define the content of the popup that appears when the user clicks the extension icon. Paste the following code into “popup.html”:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>My First Chrome Extension</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 10px;
}
</style>
</head>
<body>
<h1>Hello, world!</h1>
<p>This is a simple Chrome extension tutorial.</p>
<button id="changeColor">Change background color</button>
<script src="popup.js"></script>
</body>
</html>
Step 3: Writing background and content scripts
Create two JavaScript files named “background.js” and “content.js” in the “MyFirstExtension” folder. These files will contain the logic for your extension.
In “background.js”, paste the following code:
chrome.action.onClicked.addListener((tab) => {
chrome.scripting.executeScript({
target: { tabId: tab.id },
files: ["content.js"],
});
});
This code listens for a click event on the extension icon and executes the “content.js” script when it is clicked.
In “content.js”, paste the following code:
function getRandomColor() {
const letters = '0123456789ABCDEF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
chrome.runtime.onMessage.addListener((request, sender, sendResponse) => {
if (request.message === 'changeBackground') {
document.body.style.backgroundColor = getRandomColor();
}
});
This code defines a getRandomColor() function that generates a random hex color code. It then sets the background color of the current web page to the generated color.
Step 4: Implementing the popup script
Create a new JavaScript file named “popup.js” in the “MyFirstExtension” folder. This file will contain the logic for the popup’s “Change background color” button.
In “popup.js”, paste the following code:
document.getElementById("changeColor").addEventListener("click", () => {
chrome.tabs.query({ active: true, currentWindow: true }, (tabs) => {
chrome.scripting.executeScript(
{
target: { tabId: tabs[0].id },
files: ["content.js"],
},
() => {
chrome.tabs.sendMessage(tabs[0].id, { message: "changeBackground" });
}
);
});
});
This code listens for a click event on the “Change background color” button, and when the button is clicked, it injects the “content.js” script into the active tab and sends a message to the content script to change the background color of the web page.
Step 5: Loading and testing the extension
To load your extension in Chrome, follow these steps:
- Open Chrome and navigate to chrome://extensions/.
- Enable the “Developer mode” toggle in the top right corner.
- Click the “Load unpacked” button and select the “MyFirstExtension” folder.
Your extension should now appear in the list of extensions, and its icon should be visible in the Chrome toolbar. Click on the icon to open the popup, and click the “Change background color” button to change the background color of the current web page.
Adding Your Extension to the Chrome Web Store
After developing and testing your Chrome extension, the next step is to publish it on the Chrome Web Store to make it available for users to discover, download, and install. Follow these steps to add your extension to the Chrome Web Store:
Step 1: Prepare your extension package
Before uploading your extension, you need to create a ZIP file containing all the files in your “MyFirstExtension” folder, including the manifest file, HTML files, JavaScript files, CSS files, and icons.
To create a ZIP file:
- Select all the files in the “MyFirstExtension” folder.
- Right-click on the selected files and choose “Send to” > “Compressed (zipped) folder” (Windows) or “Compress” (macOS).
- Rename the generated ZIP file to something descriptive, such as “MyFirstExtension.zip”.
Step 2: Create a developer account
To publish extensions on the Chrome Web Store, you need a Google developer account. If you don’t already have one, follow these steps to create one:
- Visit the Chrome Web Store Developer Dashboard (https://chrome.google.com/webstore/developer/dashboard).
- Sign in with your Google account or create a new one.
- Click the “Create Developer Account” button and follow the on-screen instructions to complete the registration process, including paying a one-time registration fee.
Step 3: Upload your extension
After setting up your developer account, you can now upload your extension to the Chrome Web Store:
- Go to the Chrome Web Store Developer Dashboard.
- Click the “New item” button to start the upload process.
- Click the “Choose file” button and select the “MyFirstExtension.zip” file you created earlier.
- Wait for the file to upload. After a successful upload, you’ll be redirected to the extension’s “Edit” page.
Step 4: Fill in extension details
On the “Edit” page, you need to provide the necessary information about your extension:
- Fill in the required fields such as “Detailed description,” “Category,” and “Language.”
- Upload screenshots, promotional images, or videos to showcase your extension’s features.
- Provide a support email or website for users to contact you in case of issues or questions.
- Set the extension’s visibility (public or unlisted) and regions where it will be available.
- Specify additional information like privacy policy URL and content rating if necessary.
Step 5: Publish your extension
After filling in the extension details, click the “Submit for review” button at the bottom of the “Edit” page. Your extension will be submitted to the Chrome Web Store team for review.
The review process may take several days or even weeks, depending on various factors. Once your extension is approved, it will become publicly available on the Chrome Web Store, and users can search, download, and install it.
Conclusion
Congratulations! You have successfully created and published your first Chrome extension. With this basic knowledge, you can now explore more advanced features and create more complex extensions. Chrome extensions are a powerful way to enhance browser functionality and improve user experience, so don’t hesitate to experiment and learn more. Happy coding!
References
https://developer.chrome.com/docs/extensions/mv3/getstarted/ https://developer.chrome.com/docs/webstore/publish/
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us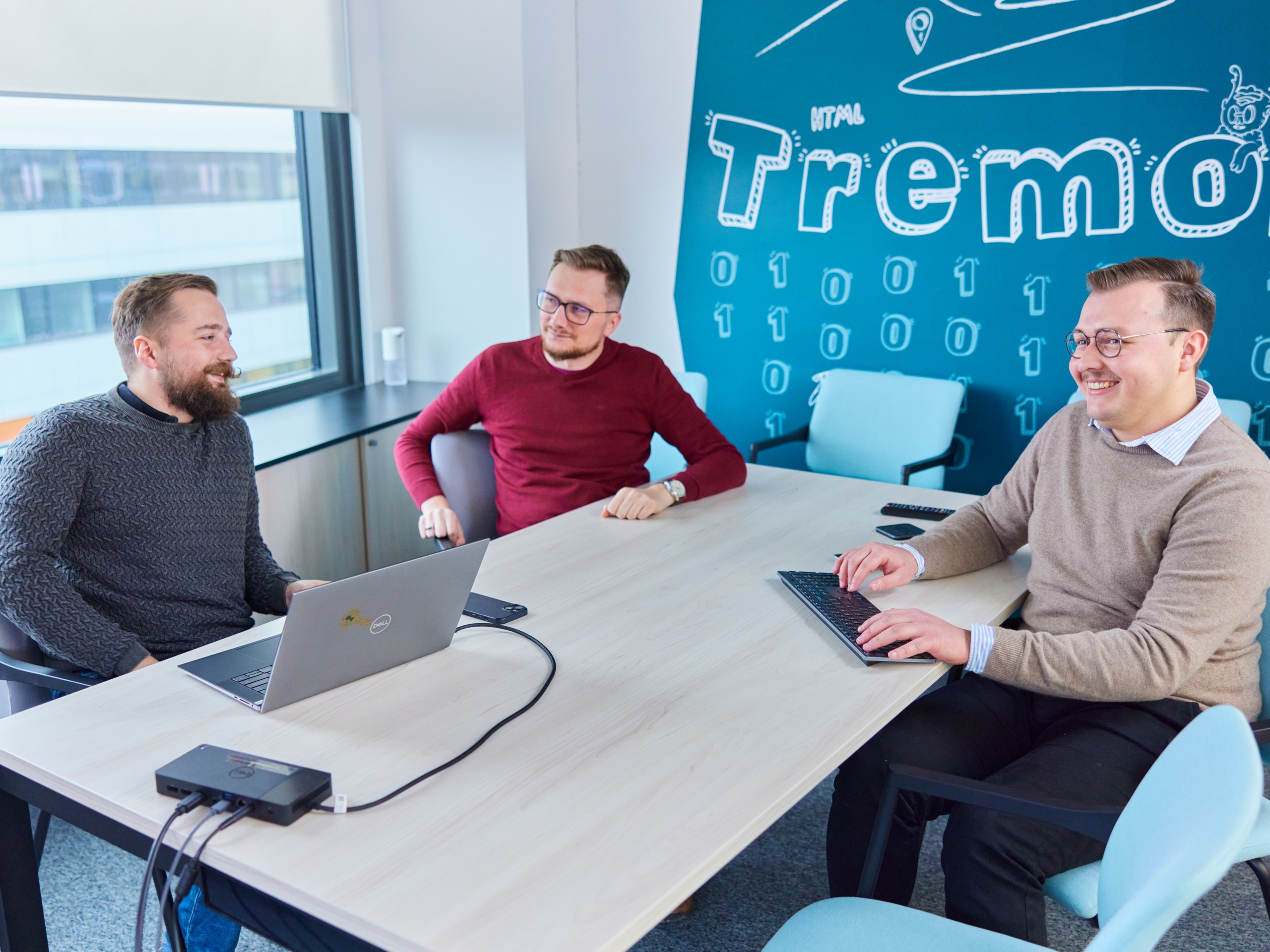
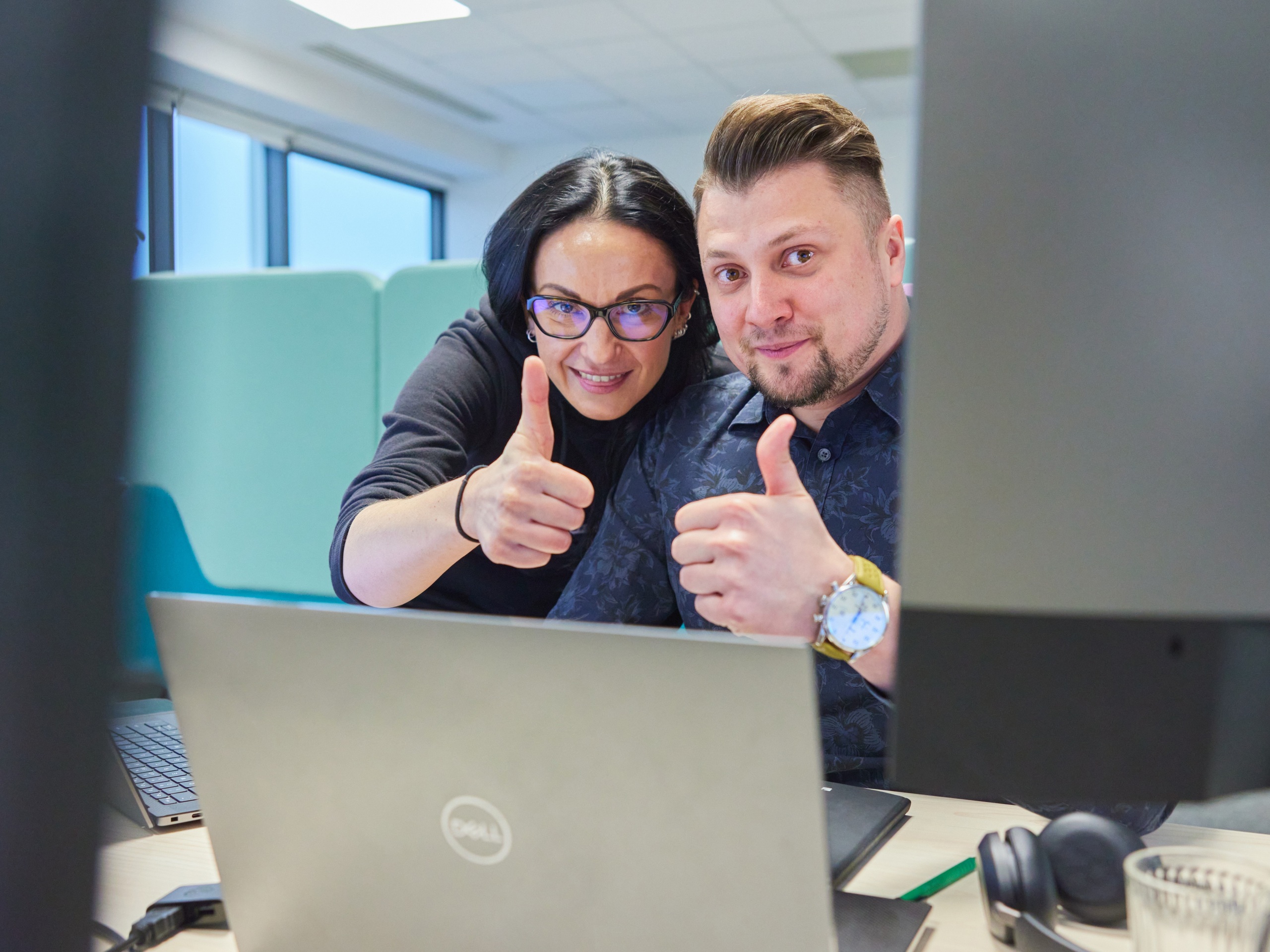
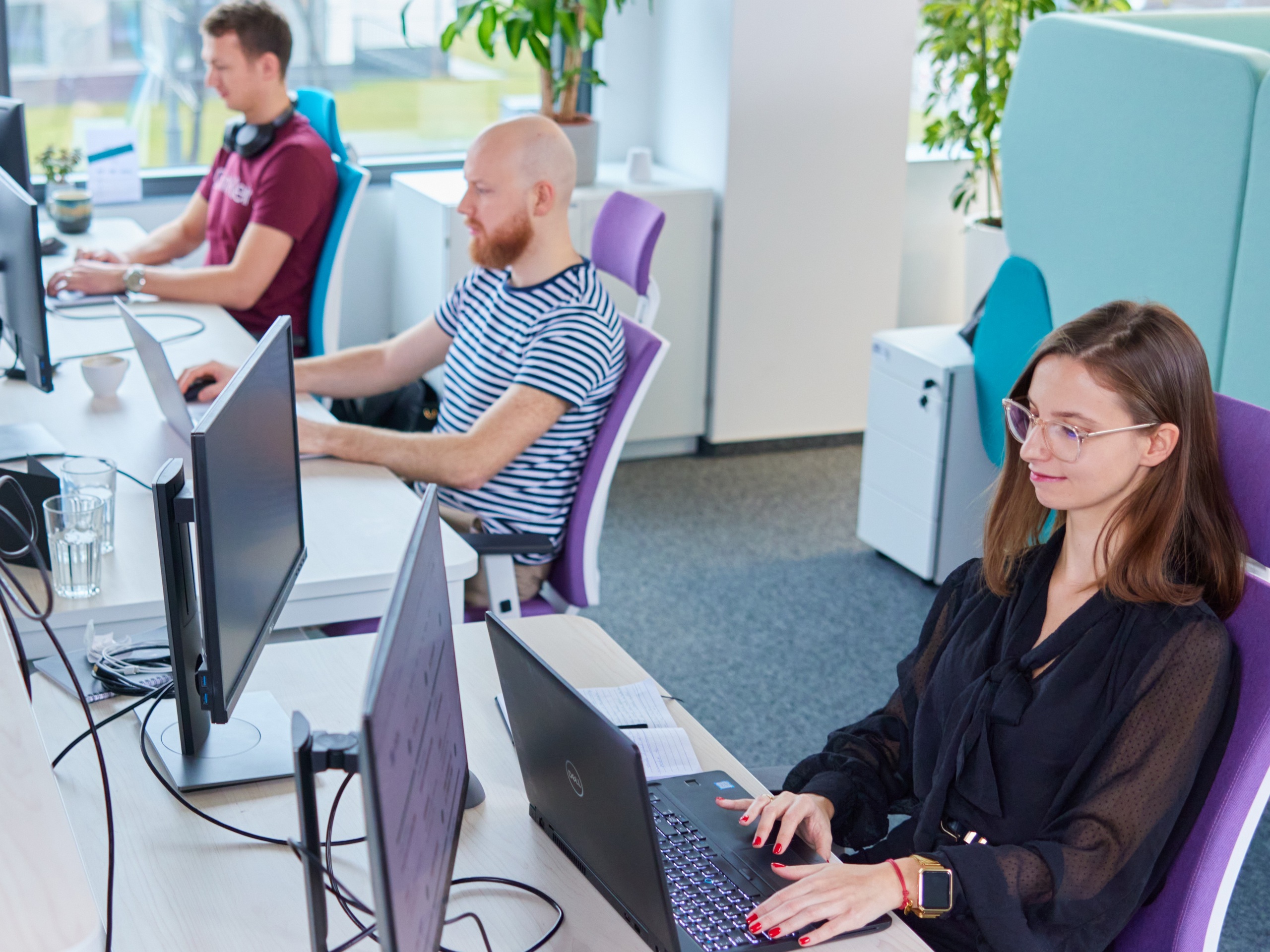
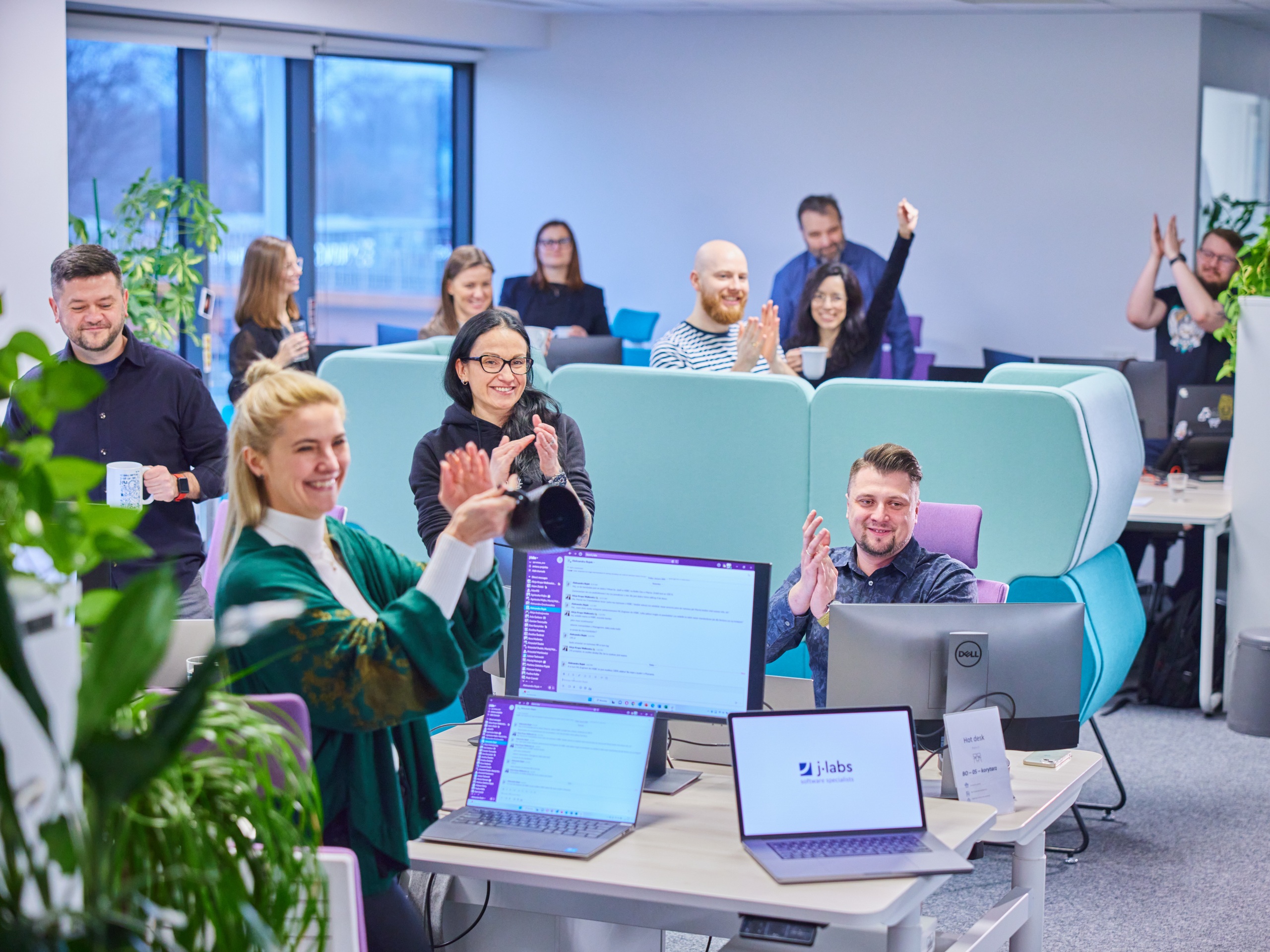