Navigating Numerical Algorithms with Java: A Journey into Mathematical Problems Solving
Introduction
Numerical algorithms form the backbone of modern scientific and engineering computations, facilitating the solution of complex mathematical problems efficiently. In this article, we embark on a journey through the realm of numerical algorithms, exploring their significance, principles, and practical implementations using the Java programming language. From basic operations to advanced numerical methods, we uncover how these algorithms underpin various real-world applications.
The Significance of Numerical Algorithms
Numerical algorithms are essential tools in solving mathematical problems that may not have closed-form solutions. They enable us to approximate solutions to intricate equations, perform calculations with real-world data, and model complex phenomena. From physics simulations to financial analysis, numerical algorithms play a pivotal role in diverse fields.
Euclidean Algorithm – The Greatest Common Divisor (GCD)
The Euclidean Algorithm, also known as the Greatest Common Divisor (GCD) algorithm, is a fundamental algorithm used to find the greatest common divisor of two integers. The greatest common divisor of two numbers is the largest positive integer that divides both numbers without leaving a remainder. The Euclidean Algorithm efficiently computes the GCD by repeatedly taking the remainder of division until the remainder becomes zero.
Here’s an implementation of the Euclidean Algorithm in Java:
public class EuclideanAlgorithm {
public static int gcd(int a, int b) {
while (b != 0) {
int remainder = a % b;
a = b;
b = remainder;
}
return a;
}
public static void main(String[] args) {
int num1 = 35;
int num2 = 15;
int result = gcd(num1, num2);
System.out.println("GCD of " + num1 + " and " + num2 + " is: " + result);
}
}
Output:
GCD of 35 and 15 is: 5
In this example, the gcd
method implements the Euclidean Algorithm. It repeatedly calculates the remainder of the division of a
by b
, and then updates a
to be b
and b
to be the remainder. This process continues until b
becomes zero. At this point, the value of a
will be the greatest common divisor of the two original numbers.
The main method demonstrates how to use the gcd
function to find the GCD of two numbers (35 and 15 in this case).
The Euclidean Algorithm is widely used in various mathematical and algorithmic contexts, such as cryptography, simplifying fractions, and optimizing algorithms. Its simplicity and efficiency make it an essential tool in computational mathematics.
Prime Number Generation (Sieve of Eratosthenes)
Prime number generation is the process of finding all prime numbers within a given range. The Sieve of Eratosthenes is a well-known algorithm for generating prime numbers efficiently. It works by iteratively marking the multiples of each prime number starting from 2, and the unmarked numbers left are primes.
Here’s an implementation of the Sieve of Eratosthenes in Java:
public class SieveOfEratosthenes {
public static void sieveOfEratosthenes(int n) {
boolean[] isPrime = new boolean[n + 1];
Arrays.fill(isPrime, true);
for (int i = 2; i * i <= n; i++) {
if (isPrime[i]) {
for (int j = i * i; j <= n; j += i) {
isPrime[j] = false;
}
}
}
System.out.println("Prime numbers in the range 2 to " + n + ":");
for (int i = 2; i <= n; i++) {
if (isPrime[i]) {
System.out.print(i + " ");
}
}
}
public static void main(String[] args) {
int n = 100;
sieveOfEratosthenes(n);
}
}
Output:
Prime numbers in the range 2 to 100:
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
In this example, the sieveOfEratosthenes
method implements the Sieve of Eratosthenes algorithm. It uses a boolean array isPrime
to mark whether a number is prime or not. The algorithm iterates through numbers starting from 2 up to the square root of n
, marking the multiples of each prime number as non-prime. The unmarked numbers left in the array are prime numbers.
The main method demonstrates how to use the sieveOfEratosthenes
function to generate prime numbers in the range from 2 to 100.
The Sieve of Eratosthenes is a highly efficient algorithm for generating prime numbers and has a time complexity of approximately O(n*log (log (n))), making it suitable for generating prime numbers within a large range.
Factorial Calculation
Factorial calculation involves finding the product of all positive integers from 1 up to a given number. It’s denoted by the symbol n!
and is used in various mathematical and combinatorial contexts.
Here’s an implementation of factorial calculation in Java:
public class FactorialCalculation {
public static long factorialRecursive(int n) {
if (n == 0 || n == 1) {
return 1;
}
return n * factorialRecursive(n - 1);
}
public static long factorialLoop(int n) {
long factorial = 1;
for (int i = 2; i <= n; i++) {
factorial = factorial * i;
}
return factorial;
}
public static long factorialStreams(int n) {
return LongStream.rangeClosed(1, n)
.reduce(1, (long x, long y) -> x * y);
}
public static void main(String[] args) {
int n = 5;
long factorialRecursive = factorialRecursive(n);
long factorialLoop = factorialLoop(n);
long factorialStreams = factorialStreams(n);
System.out.println("Factorial of " + n + " computed recursively is: " + factorialRecursive);
System.out.println("Factorial of " + n + " computed by loop is: " + factorialLoop);
System.out.println("Factorial of " + n + " computed by Stream is: " + factorialStreams);
}
}
Output:
Factorial of 5 computed recursively is: 120
Factorial of 5 computed by loop is: 120
Factorial of 5 computed by Stream is: 120
In this example, the FactorialCalculation
class implements three factorial calculation methods: using recursion, loop and Java Streams. The base cases are when n
is 0 or 1, in which case the factorial is 1. For other values of n
, the methods calculate the factorial in different ways.
The main method demonstrates how to use the factorial function to calculate the factorial of a number (5 in this case).
Factorial calculation is commonly used in various mathematical and statistical contexts, such as permutations, combinations, and probability calculations. It’s also used in algorithms and mathematical proofs.
Binary Exponentiation
Binary Exponentiation, also known as Exponentiation by Squaring, is an efficient algorithm used to calculate the power of a number raised to an integer exponent. It’s particularly useful when dealing with large numbers, as it reduces the number of multiplications required compared to naive exponentiation.
Here’s an implementation of Binary Exponentiation in Java:
public static long binaryExponentiation(long base, int exponent) {
if (exponent == 0) {
return 1;
}
long result = binaryExponentiation(base, exponent / 2);
if (exponent % 2 == 0) {
return result * result;
} else {
return base * result * result;
}
}
In this example, the binaryExponentiation
method implements the Binary Exponentiation algorithm using recursion. It calculates the power of the base raised to the exponent. The base cases are when the exponent is 0 (the result is 1) or when it’s even, in which case the result is squared. If the exponent is odd, the result is multiplied by the base.
Binary Exponentiation is highly efficient and reduces the number of multiplications required to calculate the power of a number. It’s commonly used in algorithms involving modular arithmetic, cryptography, and optimization problems.
Primality testing
Primality testing is the process of determining whether a given number is prime or composite (not prime). The Miller-Rabin primality test is a probabilistic algorithm that provides a way to test if a number is likely to be prime. It’s based on the properties of modular arithmetic and uses random numbers to make a probabilistic determination of primality.
Here’s an overview of how the Miller-Rabin algorithm works:
- Preprocessing:
- Express the given number as (2^r) * d + 1, where d is an odd integer.
- Witness Testing:
- Select a random integer a between 2 and n – 2, where n is the number being tested.
- Calculate x = a^d mod n.
- Check Conditions:
- If x is 1 or n – 1, continue with the next iteration.
- For each r in the range [1, r – 1]:
- Calculate x = x^2 mod n.
- If x is n – 1, continue with the next iteration.
- If no condition is met, the number is composite.
- Repeat:
- Repeat the above steps for the desired number of iterations (witnesses).
- If the number passes the test for all witnesses, it’s likely to be prime. Otherwise, it’s composite.
Here’s an implementation of the Miller-Rabin primality test in Java:
public class MillerRabinPrimalityTest {
public static boolean isProbablePrime(int n, int k) {
if (n <= 1) {
return false;
}
if (n <= 3) {
return true;
}
// Write n as (2^r) * d + 1
int r = 0;
int d = n - 1;
while (d % 2 == 0) {
r++;
d /= 2;
}
// Witness loop
for (int i = 0; i < k; i++) {
int a = 2 + new Random().nextInt(n - 3);
int x = power(a, d, n);
if (x == 1 || x == n - 1) {
continue;
}
for (int j = 0; j < r - 1; j++) {
x = (x * x) % n;
if (x == n - 1) {
break;
}
}
if (x != n - 1) {
return false;
}
}
return true;
}
public static int power(int x, int y, int p) {
int res = 1;
x = x % p;
while (y > 0) {
if (y % 2 == 1) {
res = (res * x) % p;
}
y = y >> 1;
x = (x * x) % p;
}
return res;
}
public static void main(String[] args) {
int num = 11;
int iterations = 5;
boolean isPrime = isProbablePrime(num, iterations);
if (isPrime) {
System.out.println(num + " is likely prime.");
} else {
System.out.println(num + " is not prime.");
}
}
}
Output:
11 is likely to be prime.
In this example, the isProbablePrime
method implements the Miller-Rabin primality test. It uses a specified number of iterations (k) to perform the test probabilistically. The power method calculates the modular exponentiation efficiently.
The main method demonstrates how to use the isProbablePrime
function to test whether a number (11 in this case) is likely to be prime using a certain number of iterations (5 in this case).
The Miller-Rabin primality test is a probabilistic algorithm, which means that it might occasionally classify a composite number as prime (a false positive), but it never misclassifies a prime number as composite (no false negatives). The accuracy of the test increases with the number of iterations performed.
Summary
Numerical algorithms are the backbone of computational mathematics, enabling us to solve complex problems with real-world implications. From basic arithmetic to advanced simulations, these algorithms pave the way for accurate solutions in diverse fields. This article’s exploration of numerical algorithms using Java demonstrates their practical significance and equips programmers with the tools to tackle complex mathematical challenges effectively.
Reference
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us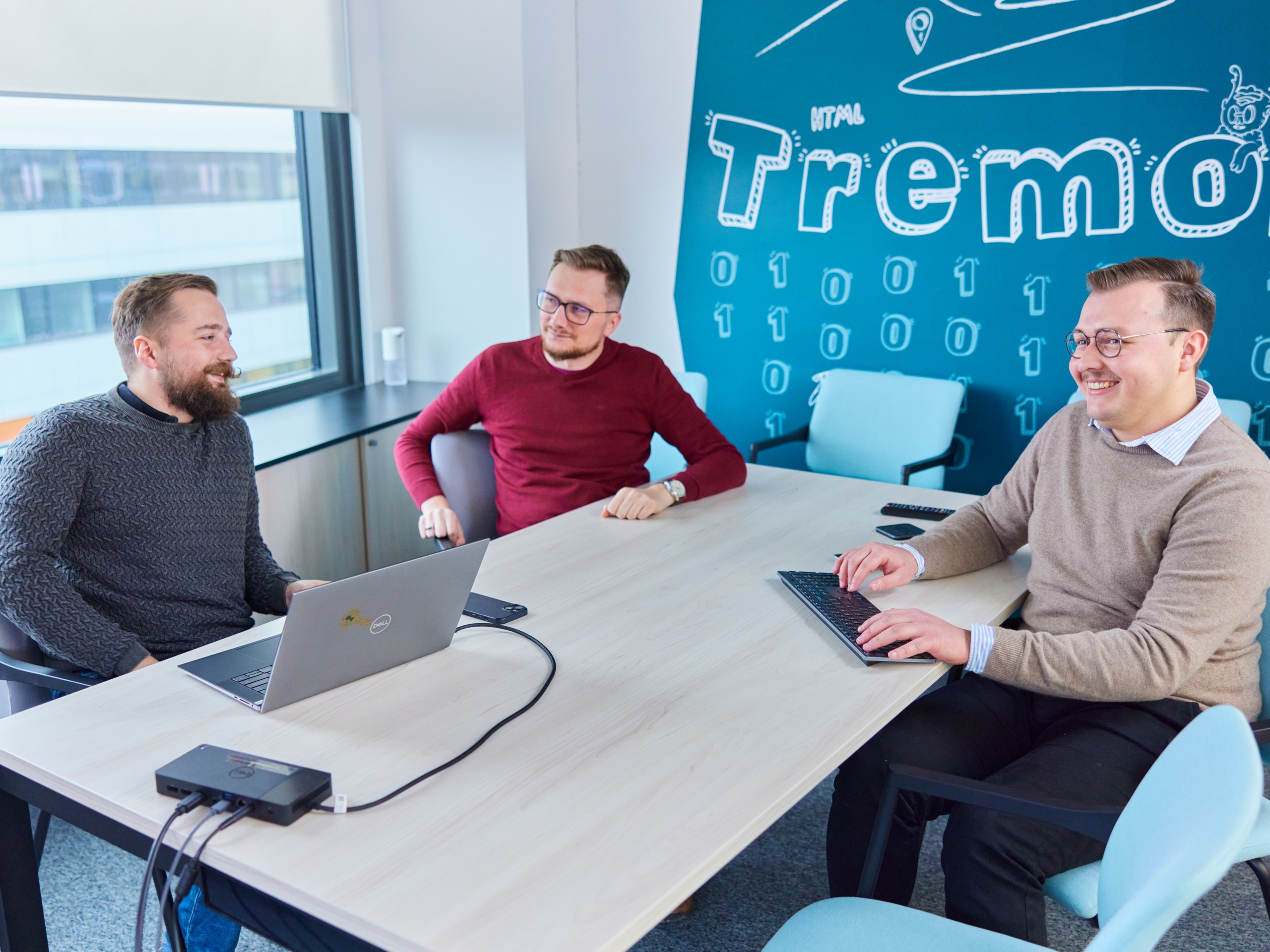
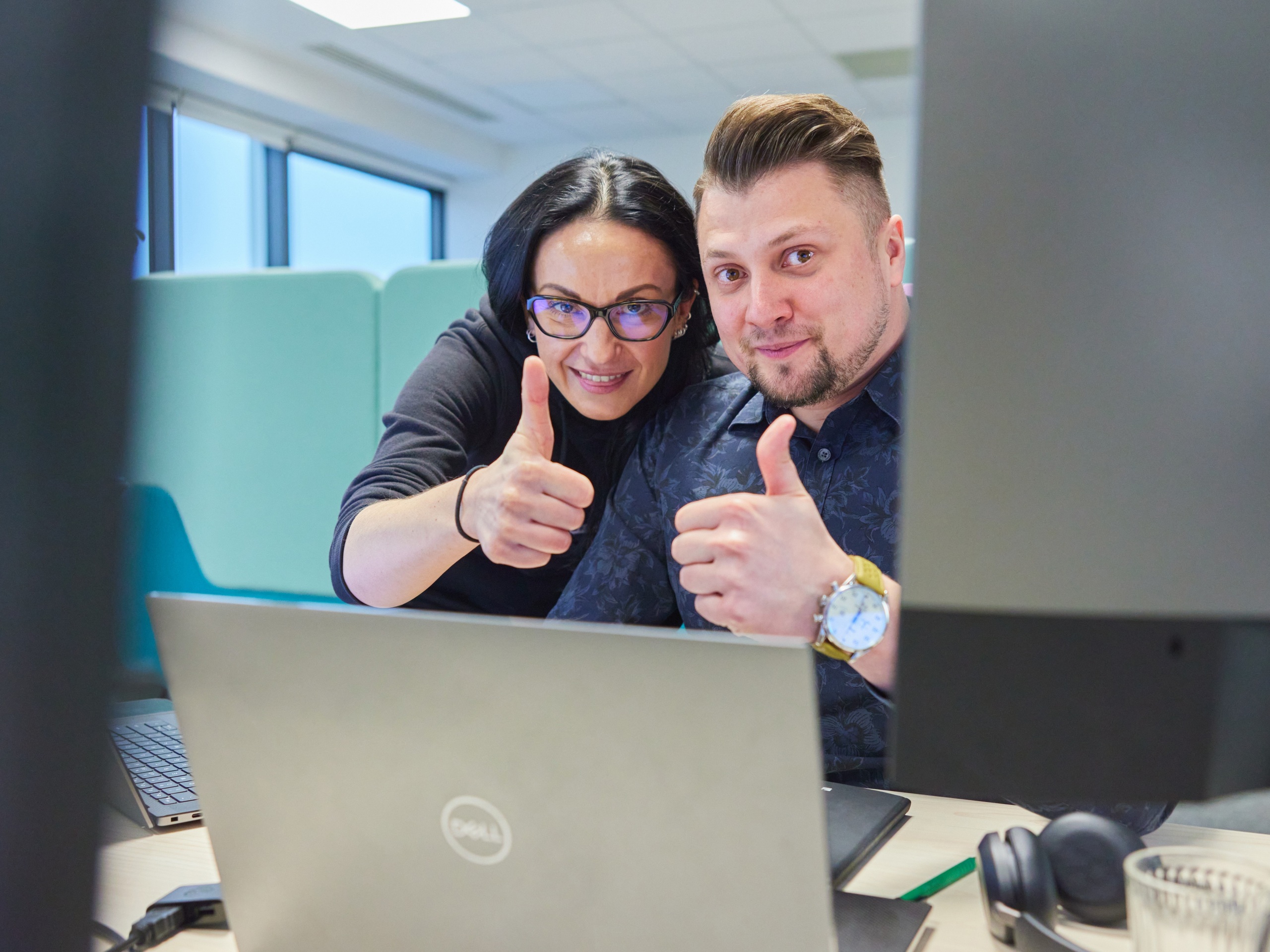
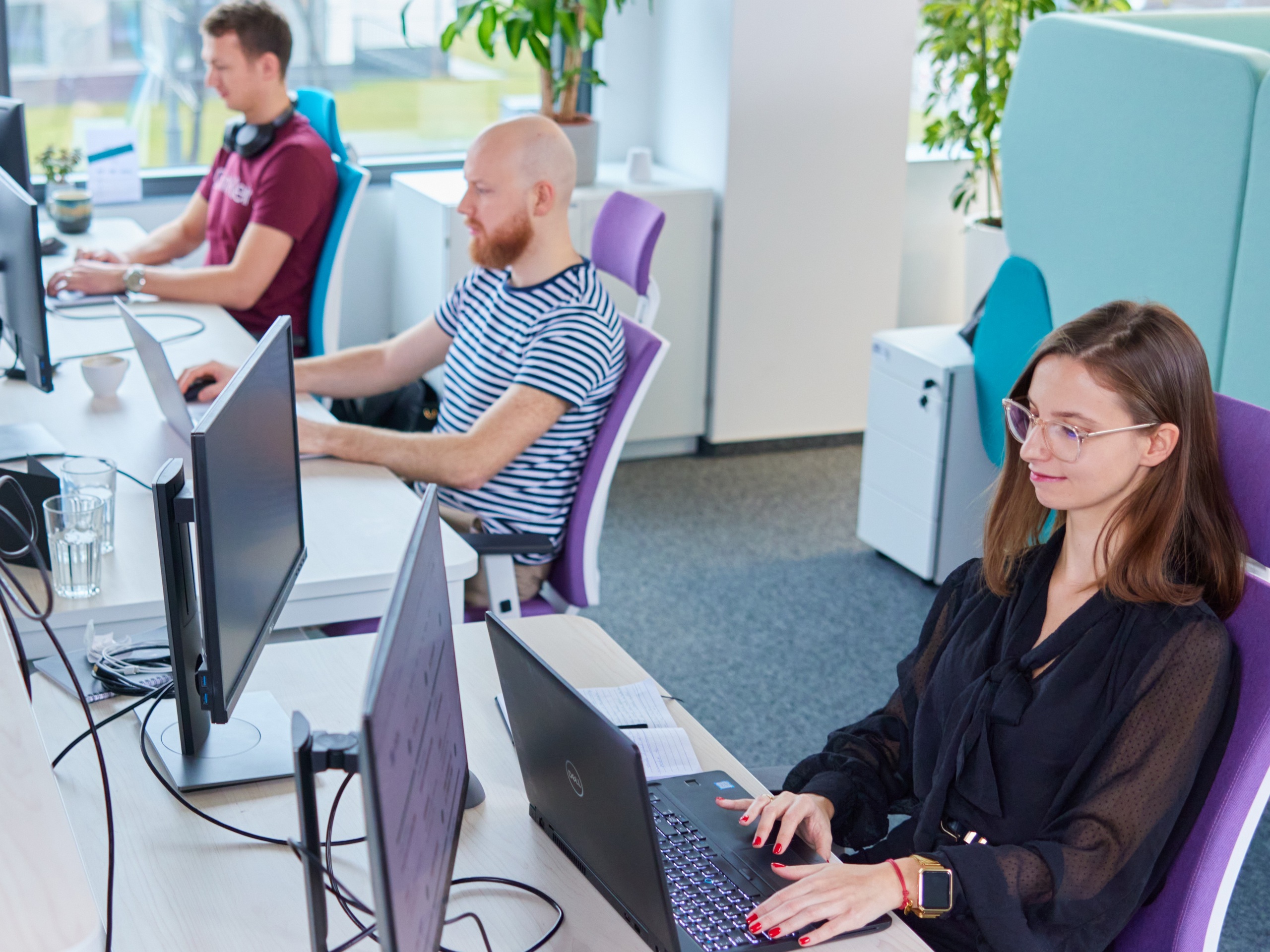
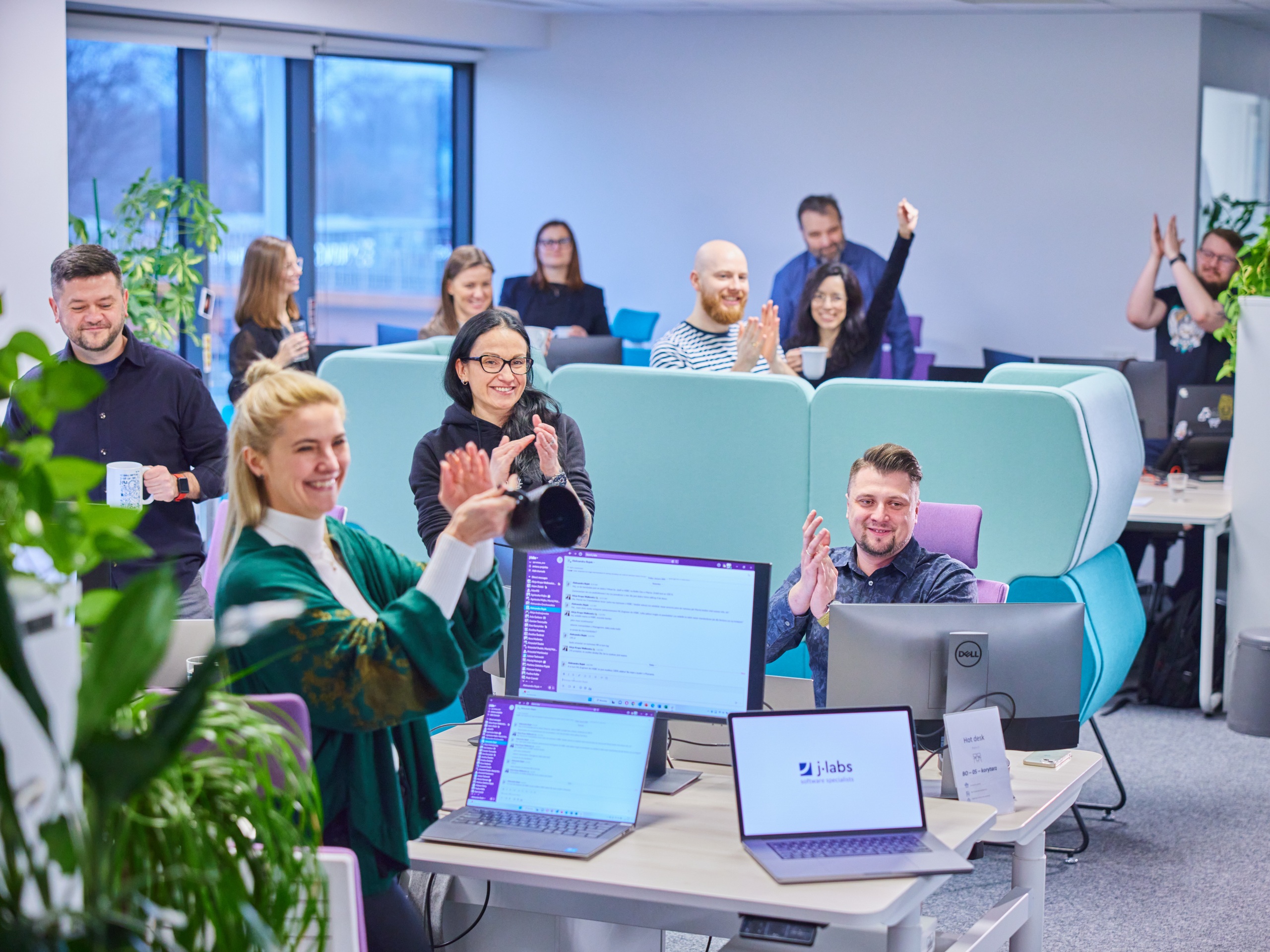